concepts::BelosSolver< T > Class Template Reference
Concepts interface to Trilinos Belos solver with different ifpack2 preconditioners and different iterative solvers. More...
#include <belosSolver.hh>
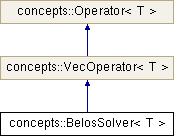
Public Types | |
typedef Cmplxtype< T >::type | c_type |
Real type of data type. More... | |
typedef Tpetra::CrsMatrix< T, int, int > | CRSmat |
typedef BelosLinProb< T, MV, OP > | LP |
typedef Tpetra::MultiVector< T, int > | MV |
typedef Tpetra::Operator< T > | OP |
typedef Realtype< T >::type | r_type |
Real type of data type. More... | |
typedef Belos::SolverManager< T, MV, OP > | solverManager |
typedef T | type |
Type of data, e.g. matrix entries. More... | |
Public Member Functions | |
BelosSolver (concepts::SparseMatrix< T > &sparse, Teuchos::RCP< const Teuchos::Comm< int > > comm) | |
Constructor for the root thread. More... | |
BelosSolver (Teuchos::RCP< const Teuchos::Comm< int > > comm) | |
Constructor for other threads wich don't know the concets::SparseMatrix. More... | |
virtual const uint | dimX () const |
Returns the size of the image space of the operator (number of rows of the corresponding matrix) More... | |
virtual const uint | dimY () const |
Returns the size of the source space of the operator (number of columns of the corresponding matrix) More... | |
Teuchos::RCP< LP > | getLinearProblem () |
Teuchos::RCP< Teuchos::ParameterList > | getPrecParam () |
Gets the parameter list for the preconditioner. More... | |
std::string | getPrecType () |
Teuchos::RCP< solverManager > | getSolverManager () |
Gets the solver via solver manager. More... | |
Teuchos::RCP< Teuchos::ParameterList > | getSolverParam () |
Get solver parameter list. More... | |
std::string | getSolverType () |
Getter for the solver type. More... | |
virtual void | operator() () |
Solving operator for other the non-root threads. More... | |
virtual void | operator() (const Function< c_type > &fncY, Function< c_type > &fncX) |
Application operator for complex function fncY . More... | |
virtual void | operator() (const Function< r_type > &fncY, Function< T > &fncX) |
Application operator for real function fncY . More... | |
void | operator() (const Matrix< c_type > &mX, Matrix< c_type > &mY) |
Application method to complex matrices. Calls apply_() More... | |
void | operator() (const Matrix< r_type > &mX, Matrix< T > &mY) |
Application method to real matrices. Calls function apply() More... | |
virtual void | operator() (const Vector< c_type > &fncY, Vector< c_type > &fncX) |
Application operator for complex function fncY . More... | |
virtual void | operator() (const Vector< r_type > &fncY, Vector< T > &fncX) |
Application operator for real vector fncY . More... | |
virtual void | operator() (const Vector< T > &fncY, Vector< T > &fncX) |
Solving operator for the root thread. More... | |
void | prepare () |
Builds the Preconditioner and the solver (sets prepare to true) More... | |
void | setPrecParam (double fill, double tol=1e-5, int absTresh=0, int relTresh=1, double relax=0) |
Set preconditoner parameter list via values. More... | |
void | setPrecParam (Teuchos::RCP< Teuchos::ParameterList > param) |
Set preconditioner parameter list via Teuchos::parameter list. More... | |
void | setPrecType (std::string type) |
Sets the preconditioner Type to one of the followings. More... | |
void | setSolverManager (Teuchos::RCP< solverManager > manager) |
Sets the solver via solver manager. More... | |
void | setSolverParam (int maxIter, int maxRestarts, double tol=1e-10, int blockSize=1, int outFreq=1) |
Set Solver parameter list via values. More... | |
void | setSolverParam (Teuchos::RCP< Teuchos::ParameterList > param) |
Set Solver parameter list via Teuchos::parameter list. More... | |
void | setSolverType (std::string type) |
Sets the solver type to one of the followings. More... | |
virtual void | show_messages () |
virtual | ~BelosSolver () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Protected Attributes | |
uint | dimX_ |
Dimension of image space and the source space. More... | |
uint | dimY_ |
Private Member Functions | |
virtual void | apply_ () |
Version for the other threads i.e. MyPID != 0. More... | |
virtual void | apply_ (const Vector< T > &fncY, Vector< T > &fncX) |
Version for the master thread i.e. MyPID = 0. More... | |
bool | createPrec_ () |
Creates Preconditioner with given params sets by setSolverParam and setSolverType. More... | |
bool | createSolver_ () |
Creates Solver with given params sets by setPrecParam and setPrecType. More... | |
bool | phasePrecInit_ (bool verbose=true) |
Builds the preconditioner. More... | |
bool | phaseSolve_ (bool verbose=true) |
Solving. More... | |
Private Attributes | |
Teuchos::RCP< const Teuchos::Comm< int > > | Comm_ |
Communicator of the mpi thread. More... | |
Teuchos::RCP< LP > | lp_ |
Linear Problem as Belos::LinearProblem. More... | |
Teuchos::RCP< Ifpack2::Preconditioner< T, int > > | prec_ |
The Preconditionier. More... | |
Teuchos::RCP< Teuchos::ParameterList > | precParam_ |
std::string | precType_ |
bool | prepared_ |
is preconditioner and all opions set More... | |
Teuchos::RCP< solverManager > | solverManager_ |
Of Type Belos::Solvermanager Handles choice of solver like CG or gmRes. More... | |
Teuchos::RCP< Teuchos::ParameterList > | solverParam_ |
Parameter for solver and preconditioner. More... | |
std::string | solverType_ |
Detailed Description
template<class T>
class concepts::BelosSolver< T >
Concepts interface to Trilinos Belos solver with different ifpack2 preconditioners and different iterative solvers.
Definition at line 32 of file belosSolver.hh.
Member Typedef Documentation
◆ c_type
|
inherited |
Real type of data type.
Definition at line 120 of file compositions.hh.
◆ CRSmat
typedef Tpetra::CrsMatrix<T, int, int> concepts::BelosSolver< T >::CRSmat |
Definition at line 38 of file belosSolver.hh.
◆ LP
typedef BelosLinProb<T, MV, OP> concepts::BelosSolver< T >::LP |
Definition at line 39 of file belosSolver.hh.
◆ MV
typedef Tpetra::MultiVector<T, int> concepts::BelosSolver< T >::MV |
Definition at line 36 of file belosSolver.hh.
◆ OP
typedef Tpetra::Operator<T> concepts::BelosSolver< T >::OP |
Definition at line 37 of file belosSolver.hh.
◆ r_type
|
inherited |
Real type of data type.
Definition at line 118 of file compositions.hh.
◆ solverManager
typedef Belos::SolverManager<T, MV, OP> concepts::BelosSolver< T >::solverManager |
Definition at line 40 of file belosSolver.hh.
◆ type
|
inherited |
Type of data, e.g. matrix entries.
Definition at line 45 of file compositions.hh.
Constructor & Destructor Documentation
◆ BelosSolver() [1/2]
concepts::BelosSolver< T >::BelosSolver | ( | concepts::SparseMatrix< T > & | sparse, |
Teuchos::RCP< const Teuchos::Comm< int > > | comm | ||
) |
Constructor for the root thread.
Definition at line 257 of file belosSolver.hh.
◆ BelosSolver() [2/2]
concepts::BelosSolver< T >::BelosSolver | ( | Teuchos::RCP< const Teuchos::Comm< int > > | comm | ) |
Constructor for other threads wich don't know the concets::SparseMatrix.
Definition at line 267 of file belosSolver.hh.
◆ ~BelosSolver()
|
inlinevirtual |
Definition at line 48 of file belosSolver.hh.
Member Function Documentation
◆ apply_() [1/2]
|
privatevirtual |
Version for the other threads i.e. MyPID != 0.
Implements concepts::VecOperator< T >.
Definition at line 193 of file belosSolver_decl.hh.
◆ apply_() [2/2]
|
privatevirtual |
Version for the master thread i.e. MyPID = 0.
Implements concepts::VecOperator< T >.
Definition at line 179 of file belosSolver_decl.hh.
◆ createPrec_()
|
private |
Creates Preconditioner with given params sets by setSolverParam and setSolverType.
Definition at line 107 of file belosSolver_decl.hh.
◆ createSolver_()
|
private |
Creates Solver with given params sets by setPrecParam and setPrecType.
Definition at line 113 of file belosSolver_decl.hh.
◆ dimX()
|
inlinevirtualinherited |
Returns the size of the image space of the operator (number of rows of the corresponding matrix)
Definition at line 93 of file compositions.hh.
◆ dimY()
|
inlinevirtualinherited |
Returns the size of the source space of the operator (number of columns of the corresponding matrix)
Definition at line 98 of file compositions.hh.
◆ getLinearProblem()
|
inline |
Definition at line 153 of file belosSolver.hh.
◆ getPrecParam()
|
inline |
Gets the parameter list for the preconditioner.
Definition at line 131 of file belosSolver.hh.
◆ getPrecType()
|
inline |
Definition at line 188 of file belosSolver.hh.
◆ getSolverManager()
|
inline |
Gets the solver via solver manager.
Definition at line 148 of file belosSolver.hh.
◆ getSolverParam()
|
inline |
Get solver parameter list.
Definition at line 98 of file belosSolver.hh.
◆ getSolverType()
|
inline |
Getter for the solver type.
Definition at line 169 of file belosSolver.hh.
◆ info()
|
protectedvirtual |
Reimplemented from concepts::VecOperator< T >.
◆ operator()() [1/8]
|
inlinevirtual |
Solving operator for other the non-root threads.
Reimplemented from concepts::Operator< T >.
Definition at line 53 of file belosSolver.hh.
◆ operator()() [2/8]
|
virtualinherited |
Application operator for complex function fncY
.
Computes fncX
= A(fncY
) where A is this operator. fncX
becomes complex.
In derived classes its enough to implement the operator() for complex Operator's. If a real counterpart is not implemented, the function fncY
is splitted into real and imaginary part and the application operator for real functions is called for each. Then the result is combined.
If in a derived class the operator() for complex Operator's is not implemented, a exception is thrown from here.
Reimplemented from concepts::Operator< T >.
◆ operator()() [3/8]
|
virtualinherited |
Application operator for real function fncY
.
Computes fncX
= A(fncY
) where A is this operator.
fncX
becomes the type of the operator, for real data it becomes real, for complex data it becomes complex.
In derived classes its enough to implement the operator() for real Operator's. If a complex counterpart is not implemented, the function fncY
is transformed to a complex function and then the application operator for complex functions is called.
If in a derived class the operator() for real Operator's is not implemented, a exception is thrown from here.
Reimplemented from concepts::Operator< T >.
◆ operator()() [4/8]
|
inherited |
Application method to complex matrices. Calls apply_()
◆ operator()() [5/8]
|
inherited |
Application method to real matrices. Calls function apply()
◆ operator()() [6/8]
|
virtualinherited |
Application operator for complex function fncY
.
Computes fncX
= A(fncY
) where A is this operator. fncX
becomes complex.
In derived classes its enough to implement the operator() for complex Operator's. If a real counterpart is not implemented, the vector fncY
is splitted into real and imaginary part and the application operator for real vectors is called for each. Then the result is combined
If in a derived class the operator() for complex Operator's i not implemented, a exception is thrown from here.
◆ operator()() [7/8]
|
virtualinherited |
Application operator for real vector fncY
.
Computes fncX
= A(fncY
) where A is this operator.
Type of fncX
becomes that of the operator, for real data it becomes real, for complex data it becomes complex.
In derived classes its enough to implement the operator() for real Operator's. If a complex counterpart is not implemented, the vector fncY
is transformed to a complex vector and then the application for complex vectors is called.
If in a derived class the operator() for real Operator's is not implemented, a exception is thrown from here.
◆ operator()() [8/8]
|
inlinevirtual |
Solving operator for the root thread.
- Parameters
-
fncY the right hand side the solution vector
Definition at line 62 of file belosSolver.hh.
◆ phasePrecInit_()
|
private |
Builds the preconditioner.
Definition at line 121 of file belosSolver_decl.hh.
◆ phaseSolve_()
|
private |
Solving.
Definition at line 146 of file belosSolver_decl.hh.
◆ prepare()
|
inline |
Builds the Preconditioner and the solver (sets prepare to true)
Definition at line 194 of file belosSolver.hh.
◆ setPrecParam() [1/2]
|
inline |
Set preconditoner parameter list via values.
- Parameters
-
fill width of the bandmatrix used for preconditioning tol tolerance for the preconditioner
Definition at line 116 of file belosSolver.hh.
◆ setPrecParam() [2/2]
|
inline |
Set preconditioner parameter list via Teuchos::parameter list.
- Parameters
-
param Parameterlist that contains the parameter for the preconditioner
Definition at line 106 of file belosSolver.hh.
◆ setPrecType()
|
inline |
Sets the preconditioner Type to one of the followings.
"DIAGONAL"
"RELAXATION"
"CHEBYSHEV"
"ILUT"
"RILUK"
Definition at line 182 of file belosSolver.hh.
◆ setSolverManager()
|
inline |
Sets the solver via solver manager.
Definition at line 139 of file belosSolver.hh.
◆ setSolverParam() [1/2]
|
inline |
Set Solver parameter list via values.
- Parameters
-
maxIter maximal number of iterations maxRestarts maximal number of restarts tol tolerence for the residuum
Definition at line 80 of file belosSolver.hh.
◆ setSolverParam() [2/2]
|
inline |
Set Solver parameter list via Teuchos::parameter list.
- Parameters
-
param Parameterlist that contains the parameters for the solver
Definition at line 70 of file belosSolver.hh.
◆ setSolverType()
|
inline |
Sets the solver type to one of the followings.
"GMRES"
"BLOCKCG"
"PSEUDOCG"
Definition at line 163 of file belosSolver.hh.
◆ show_messages()
|
inlinevirtualinherited |
Definition at line 100 of file compositions.hh.
Member Data Documentation
◆ Comm_
|
private |
Communicator of the mpi thread.
Definition at line 231 of file belosSolver.hh.
◆ dimX_
|
protectedinherited |
Dimension of image space and the source space.
Definition at line 104 of file compositions.hh.
◆ dimY_
|
protectedinherited |
Definition at line 104 of file compositions.hh.
◆ lp_
|
private |
Linear Problem as Belos::LinearProblem.
Definition at line 234 of file belosSolver.hh.
◆ prec_
|
private |
The Preconditionier.
Definition at line 244 of file belosSolver.hh.
◆ precParam_
|
private |
Definition at line 238 of file belosSolver.hh.
◆ precType_
|
private |
Definition at line 248 of file belosSolver.hh.
◆ prepared_
|
private |
is preconditioner and all opions set
Definition at line 251 of file belosSolver.hh.
◆ solverManager_
|
private |
Of Type Belos::Solvermanager Handles choice of solver like CG or gmRes.
Definition at line 241 of file belosSolver.hh.
◆ solverParam_
|
private |
Parameter for solver and preconditioner.
Definition at line 237 of file belosSolver.hh.
◆ solverType_
|
private |
Definition at line 247 of file belosSolver.hh.
The documentation for this class was generated from the following files:
- operator/belosSolver.hh
- operator/belosSolver_decl.hh