concepts::ImportMesh Class Reference
Base class for reading a mesh from a file. More...
#include <meshImport.hh>
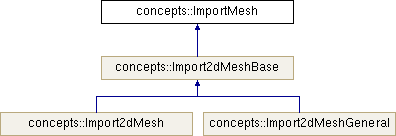
Public Member Functions | |
ImportMesh (const uint idxStart=1) | |
Constructor. More... | |
virtual | ~ImportMesh () |
Protected Member Functions | |
template<class T > | |
void | clear_ (std::vector< T * > &field) |
Deletes the content of field of pointers. More... | |
virtual void | createCell_ (const MultiIndex< 2 > &idx) throw (concepts::MissingFeature) |
Creation of geometrical cells with element mapping. More... | |
virtual void | createCell_ (const MultiIndex< 3 > &idx) throw (concepts::MissingFeature) |
virtual void | createCell_ (const MultiIndex< 4 > &idx) throw (concepts::MissingFeature) |
virtual void | createCell_ (const MultiIndex< 6 > &idx) throw (concepts::MissingFeature) |
virtual void | createCell_ (const MultiIndex< 8 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 1 > &idx) |
Creation of topological entities Vertex and Edge. More... | |
virtual void | createEntity_ (const MultiIndex< 2 > &idx) |
virtual void | createEntity_ (const MultiIndex< 3 > &idx) throw (concepts::MissingFeature) |
Creation of topological entities. More... | |
virtual void | createEntity_ (const MultiIndex< 4 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 6 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 8 > &idx) throw (concepts::MissingFeature) |
template<uint dim> | |
void | newCell_ (MultiIndex< dim > i) |
Creates topological entities and a geometrical cell. More... | |
void | readAttributes_ (const std::string &bound, const Array< bool > &dimensions) |
void | readCells_ (const std::string &elms, const Array< bool > &dimensions, const std::vector< Real3d > &vertices) |
Reads the file with cell information and creates topological entities and geometrical cells. More... | |
uint | readInts_ (const std::string &i, std::vector< int > &v) const |
Reads integers from the string. More... | |
template<class F > | |
uint | readLine_ (const std::string &i, Array< F > &a, bool first=false) const |
Reads line of numbers from the string. More... | |
Protected Attributes | |
std::vector< Connector1 * > | edg_ |
MultiArray< 2, Edge * > | Edg_ |
MultiArray< 8, Attribute > | eightAttr_ |
MultiArray< 4, Attribute > | fourAttr_ |
const uint | idxStart_ |
Starting point of indices in the files (1 or 0) More... | |
MultiArray< 1, Attribute > | oneAttr_ |
Attributes of entities. More... | |
MultiArray< 6, Attribute > | sixAttr_ |
MultiArray< 3, Attribute > | threeAttr_ |
MultiArray< 2, Attribute > | twoAttr_ |
std::vector< Vertex * > | vtx_ |
List of entities. More... | |
MultiArray< 1, Vertex * > | Vtx_ |
Array of the entities. More... | |
Detailed Description
Base class for reading a mesh from a file.
The orientation of the edges is determined by the order of the vertices in the coordinate file, i.e. the first vertex in the coordinate file is the first vertex of the edge.
Definition at line 32 of file meshImport.hh.
Constructor & Destructor Documentation
◆ ImportMesh()
|
inline |
Constructor.
- Parameters
-
idxStart Starting point of indices in the files (1 or 0)
Definition at line 37 of file meshImport.hh.
◆ ~ImportMesh()
|
virtual |
Member Function Documentation
◆ clear_()
|
protected |
Deletes the content of field
of pointers.
Definition at line 141 of file meshImport.hh.
◆ createCell_() [1/5]
|
protectedvirtual |
Creation of geometrical cells with element mapping.
Has to be implemented in derivated classes.
◆ createCell_() [2/5]
|
protectedvirtual |
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createCell_() [3/5]
|
protectedvirtual |
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createCell_() [4/5]
|
protectedvirtual |
◆ createCell_() [5/5]
|
protectedvirtual |
◆ createEntity_() [1/6]
|
protectedvirtual |
Creation of topological entities Vertex and Edge.
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createEntity_() [2/6]
|
protectedvirtual |
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createEntity_() [3/6]
|
protectedvirtual |
Creation of topological entities.
Has to be implemented in derivated classes.
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createEntity_() [4/6]
|
protectedvirtual |
Reimplemented in concepts::Import2dMeshGeneral, and concepts::Import2dMeshBase.
◆ createEntity_() [5/6]
|
protectedvirtual |
◆ createEntity_() [6/6]
|
protectedvirtual |
◆ newCell_()
|
protected |
Creates topological entities and a geometrical cell.
◆ readAttributes_()
|
protected |
◆ readCells_()
|
protected |
Reads the file with cell information and creates topological entities and geometrical cells.
◆ readInts_()
|
protected |
Reads integers from the string.
- Returns
- Number of integers read
◆ readLine_()
|
protected |
Reads line of numbers from the string.
- Parameters
-
i String a resulting array of integers first flag, if the first integer should be taken into the array
- Returns
- Number of integers read
Definition at line 123 of file meshImport.hh.
Member Data Documentation
◆ edg_
|
protected |
Definition at line 47 of file meshImport.hh.
◆ Edg_
|
protected |
Definition at line 50 of file meshImport.hh.
◆ eightAttr_
|
protected |
Definition at line 58 of file meshImport.hh.
◆ fourAttr_
|
protected |
Definition at line 56 of file meshImport.hh.
◆ idxStart_
|
protected |
Starting point of indices in the files (1 or 0)
Definition at line 43 of file meshImport.hh.
◆ oneAttr_
|
protected |
Attributes of entities.
Definition at line 53 of file meshImport.hh.
◆ sixAttr_
|
protected |
Definition at line 57 of file meshImport.hh.
◆ threeAttr_
|
protected |
Definition at line 55 of file meshImport.hh.
◆ twoAttr_
|
protected |
Definition at line 54 of file meshImport.hh.
◆ vtx_
|
protected |
List of entities.
Definition at line 46 of file meshImport.hh.
◆ Vtx_
|
protected |
Array of the entities.
Definition at line 49 of file meshImport.hh.
The documentation for this class was generated from the following file:
- geometry/meshImport.hh