concepts::UnitNd< dim > Class Template Reference
A vector of dimension dim and length 1. More...
#include <vectorsMatrices.hh>
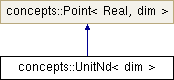
Public Member Functions | |
Real | dot (const Point< Real, dim > &b) const |
Inner product, i.e. for complex arguments: this * conjugate(b) More... | |
std::ostream & | info (std::ostream &os) const |
Real | l2 () const |
Returns the Euclidian norm of the vector. More... | |
Real | l2_2 () const |
Returns the square of the Euclidian norm of the vector. More... | |
Real | length () const |
Length of the initially given vector. More... | |
void | lincomb (const Point< Real, dim > &a, const Point< Real, dim > &b, const Real ca=1.0, const Real cb=1.0) |
Assign the vector the linear combination of a and b. More... | |
Real | linfty () const |
Returns the Maximum norm of the vector. More... | |
void | max (const Point< Real, dim > &a, const Point< Real, dim > &b) |
Sets the vector to the elementwise maximum of a and b. More... | |
void | min (const Point< Real, dim > &a, const Point< Real, dim > &b) |
Sets the vector to the elementwise minimum of a and b. More... | |
operator const Real * () const | |
Returns a pointer to the data. More... | |
operator Real * () | |
Returns a pointer to the data. More... | |
Point< Cmplx, dim > | operator* (const Cmplx n) const |
Scaling operator with a complex number. More... | |
Real | operator* (const Point< Real, dim > &b) const |
Inner product. More... | |
Point< Real, dim > | operator* (const Real n) const |
Scaling operator with a real number. More... | |
Real | operator* (const UnitNd< dim > &b) const |
Point< Real, dim > & | operator*= (const G n) |
Scaling operator. More... | |
Point< Real, dim > | operator+ (const Point< Real, dim > &p) const |
Addition operator. More... | |
Point< Real, dim > & | operator+= (const Point< Real, dim > &p) |
Addition operator. More... | |
Point< Real, dim > | operator- (const Point< Real, dim > &p) const |
Subtraction operator. More... | |
Point< Real, dim > & | operator-= (const Point< Real, dim > &p) |
Subtraction operator. More... | |
Point< Cmplx, dim > | operator/ (const Cmplx n) const |
Unscaling operator with a complex number. More... | |
Point< Real, dim > | operator/ (const Real n) const |
Unscaling operator with a real number. More... | |
Point< Real, dim > & | operator/= (const G n) |
Unscaling operator. More... | |
Real & | operator[] (const uint i) |
Index operator. More... | |
const Real & | operator[] (const uint i) const |
Index operator. More... | |
Real | operator^ (const Point< Real, 2 > &b) const |
Outer product (for 2D) More... | |
Point< Real, 3 > | operator^ (const Point< Real, 3 > &b) const |
Outer product (for 3D) More... | |
Point< Real, dim > & | ortho () |
Rotates the vector by 90 degrees (clockwise) (only 2D) More... | |
Point< Real, dim > | ortho () const |
Returns a by 90 degrees (clockwise) rotated vector (only 2D) More... | |
Point< Real, dim > & | ortho (const Point< Real, dim > &a) |
Change vector to the by 90 degrees (clockwise) rotated vector a (only 2D) More... | |
Point< Real, dim > & | scale (const Point< Real, dim > &n) |
Scaling. More... | |
UnitNd () | |
Constructor. Initializes all elements to 0. More... | |
UnitNd (const Point< Real, dim > &u) | |
Constructor for a point. More... | |
UnitNd (Real x, Real y, Real z) | |
Constructor for at most 3D. More... | |
Private Attributes | |
Real | data_ [dim] |
Real | len_ |
Detailed Description
template<uint dim>
class concepts::UnitNd< dim >
A vector of dimension dim and length 1.
Definition at line 276 of file vectorsMatrices.hh.
Constructor & Destructor Documentation
◆ UnitNd() [1/3]
|
inline |
Constructor. Initializes all elements to 0.
Definition at line 281 of file vectorsMatrices.hh.
◆ UnitNd() [2/3]
|
inline |
Constructor for a point.
Definition at line 292 of file vectorsMatrices.hh.
◆ UnitNd() [3/3]
|
inline |
Constructor for at most 3D.
Definition at line 298 of file vectorsMatrices.hh.
Member Function Documentation
◆ dot()
|
inherited |
Inner product, i.e. for complex arguments: this * conjugate(b)
◆ info()
|
inherited |
◆ l2()
|
inlineinherited |
Returns the Euclidian norm of the vector.
Definition at line 182 of file vectorsMatrices.hh.
◆ l2_2()
|
inherited |
Returns the square of the Euclidian norm of the vector.
◆ length()
|
inline |
Length of the initially given vector.
Definition at line 288 of file vectorsMatrices.hh.
◆ lincomb()
|
inherited |
Assign the vector the linear combination of a and b.
◆ linfty()
|
inherited |
Returns the Maximum norm of the vector.
◆ max()
|
inherited |
Sets the vector to the elementwise maximum of a and b.
◆ min()
|
inherited |
Sets the vector to the elementwise minimum of a and b.
◆ operator const Real *()
|
inlineinherited |
Returns a pointer to the data.
Definition at line 101 of file vectorsMatrices.hh.
◆ operator Real *()
|
inlineinherited |
Returns a pointer to the data.
Definition at line 99 of file vectorsMatrices.hh.
◆ operator*() [1/4]
|
inlineinherited |
Scaling operator with a complex number.
Definition at line 148 of file vectorsMatrices.hh.
◆ operator*() [2/4]
|
inherited |
Inner product.
◆ operator*() [3/4]
|
inlineinherited |
Scaling operator with a real number.
Definition at line 153 of file vectorsMatrices.hh.
◆ operator*() [4/4]
|
inherited |
◆ operator*=()
|
inlineinherited |
Scaling operator.
Definition at line 134 of file vectorsMatrices.hh.
◆ operator+()
|
inlineinherited |
Addition operator.
Definition at line 110 of file vectorsMatrices.hh.
◆ operator+=()
|
inlineinherited |
Addition operator.
Definition at line 104 of file vectorsMatrices.hh.
◆ operator-()
|
inlineinherited |
Subtraction operator.
Definition at line 121 of file vectorsMatrices.hh.
◆ operator-=()
|
inlineinherited |
Subtraction operator.
Definition at line 115 of file vectorsMatrices.hh.
◆ operator/() [1/2]
|
inlineinherited |
Unscaling operator with a complex number.
Definition at line 159 of file vectorsMatrices.hh.
◆ operator/() [2/2]
|
inlineinherited |
Unscaling operator with a real number.
Definition at line 164 of file vectorsMatrices.hh.
◆ operator/=()
|
inlineinherited |
Unscaling operator.
Definition at line 142 of file vectorsMatrices.hh.
◆ operator[]() [1/2]
|
inlineinherited |
Index operator.
Definition at line 94 of file vectorsMatrices.hh.
◆ operator[]() [2/2]
|
inlineinherited |
Index operator.
Definition at line 90 of file vectorsMatrices.hh.
◆ operator^() [1/2]
|
inherited |
Outer product (for 2D)
◆ operator^() [2/2]
|
inherited |
Outer product (for 3D)
◆ ortho() [1/3]
|
inherited |
Rotates the vector by 90 degrees (clockwise) (only 2D)
◆ ortho() [2/3]
|
inherited |
Returns a by 90 degrees (clockwise) rotated vector (only 2D)
◆ ortho() [3/3]
Change vector to the by 90 degrees (clockwise) rotated vector a
(only 2D)
◆ scale()
|
inlineinherited |
Scaling.
Definition at line 170 of file vectorsMatrices.hh.
Member Data Documentation
◆ data_
|
privateinherited |
Definition at line 209 of file vectorsMatrices.hh.
◆ len_
|
private |
Definition at line 277 of file vectorsMatrices.hh.
The documentation for this class was generated from the following file:
- basics/vectorsMatrices.hh