eigensolver::ArPackppGen< F, G, H > Class Template Referenceabstract
EigenSolver for complex, general eigenproblems. More...
#include <arpackpp.hh>
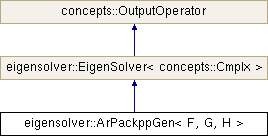
Public Member Functions | |
ArPackppGen (concepts::Operator< F > &OP, concepts::Operator< G > &A, concepts::Operator< H > &B, int kmax=1, char *which=(char *) "LM", Real tol=0.0, int maxiter=300) | |
Regular mode constructor. More... | |
ArPackppGen (concepts::Operator< F > &OP, concepts::Operator< G > &A, concepts::Operator< H > &B, int kmax=1, concepts::Cmplx sigma=0.0, Real tol=0.0, int maxiter=300) | |
Shift and invert mode constructor. More... | |
virtual uint | converged () const |
Returns the number of converged eigenpairs. More... | |
virtual uint | converged () const=0 |
Returns the number of converged eigen pairs. More... | |
virtual concepts::Array< concepts::Vector< Cmplx > * > & | getEF () |
Getter for the computed eigenvectors. More... | |
virtual const concepts::Array< Cmplx > & | getEV () |
Getter for the computed eigenvalues. More... | |
concepts::Array< Cmplx > | getRESID () |
Returns the RESID vector. More... | |
virtual uint | iterations () const |
Returns the actual number of Arnoldi iterations. More... | |
virtual uint | iterations () const=0 |
Returns the number of iterations. More... | |
virtual | ~ArPackppGen () |
Deconstructor. More... | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
void | compute_ () |
Computes and stores the eigenpairs. More... | |
Private Attributes | |
ArpackOperatorWrapper< F, G, H > | aow_ |
Wrapper. More... | |
ARCompGenEig< Real, ArpackOperatorWrapper< F, G, H >, ArpackOperatorWrapper< F, G, H > > | arpackSolver_ |
Arpack solver. More... | |
bool | computed_ |
Whether or not eigenpairs have already been computed. More... | |
uint | convEigenvalues_ |
Number of converged eigenpairs. More... | |
concepts::Array< Cmplx > | eigenValues_ |
References into storage for eigenvalues. More... | |
concepts::Array< concepts::Vector< Cmplx > * > | eigenVectors_ |
References into storage for eigenvectors. More... | |
uint | iter_ |
Number of Arnoldi iterations. More... | |
ArPack< Real >::modus | modus_ |
Mode of the Arpack solver. More... | |
char * | whichEV_ |
Identifier indicating which eigenvalues should be calculated. More... | |
Detailed Description
template<class F, class G, class H = concepts::Real>
class eigensolver::ArPackppGen< F, G, H >
EigenSolver for complex, general eigenproblems.
Solves problems of the form with complex matrix A and real, symmetric, positive definite matrix B.
Definition at line 541 of file arpackpp.hh.
Constructor & Destructor Documentation
◆ ArPackppGen() [1/2]
|
inline |
Shift and invert mode constructor.
- Parameters
-
OP multiplication operator, OP * x = inv(A-sigma*B) * x A matrix of the left hand side B matrix of the right hand side kmax number of eigenpairs to be computed, default 1 sigma complex-valued shift for the shift-invert mode, default 0.0 tol convergence tolerance for the eigenpairs, default 0.0 (is replaced by LAPACK's DLAMCH
('EPS'))maxiter maximum number of Arnoldi iterations allowed, default 300
- Warning
B
has to be real, symmetric and positive definite.-
kmax
has to be larger than or equal to 1 and smaller than or equal to the dimension of the matrix minus 2.
Definition at line 557 of file arpackpp.hh.
◆ ArPackppGen() [2/2]
|
inline |
Regular mode constructor.
- Parameters
-
OP multiplication operator, OP * x = inv(B) * x A matrix of the left hand side B matrix of the right hand side kmax number of eigenpairs to be computed, default 1 which defines which sort of eigenvalues should be computed, i.e. eigenvalues of largest magnitude ("LM"), smallest magnitude ("SM"), largest real part ("LR"), smallest real part ("SR"), largest imaginary part ("LI") or smallest imaginary part ("SI"), default "LM" tol convergence tolerance for the eigenpairs, default 0.0 (is replaced by LAPACK's DLAMCH
('EPS'))maxiter maximum number of Arnoldi iterations allowed, default 300
- Warning
B
has to be real, symmetric and positive definite.-
kmax
has to be larger than or equal to 1 and smaller than or equal to the dimension of the matrix minus 2.
Definition at line 603 of file arpackpp.hh.
◆ ~ArPackppGen()
|
inlinevirtual |
Deconstructor.
Definition at line 632 of file arpackpp.hh.
Member Function Documentation
◆ compute_()
|
inlineprivate |
Computes and stores the eigenpairs.
Definition at line 698 of file arpackpp.hh.
◆ converged() [1/2]
|
inlinevirtual |
Returns the number of converged eigenpairs.
Definition at line 665 of file arpackpp.hh.
◆ converged() [2/2]
|
pure virtualinherited |
Returns the number of converged eigen pairs.
Implemented in eigensolver::ArPackppGen< typename eigensolver::OperatorType< F, concepts::Real >::type, F, concepts::Real >, eigensolver::ArPackppStd< typename eigensolver::OperatorType< Cmplx, Real >::type >, and eigensolver::ArPackppStd< F >.
◆ getEF()
|
inlinevirtual |
Getter for the computed eigenvectors.
Computes eigenvalues and eigenvectors if they have not yet been computed.
Implements eigensolver::EigenSolver< concepts::Cmplx >.
Definition at line 653 of file arpackpp.hh.
◆ getEV()
|
inlinevirtual |
Getter for the computed eigenvalues.
Computes eigenvalues and eigenvectors if they have not yet been computed.
Implements eigensolver::EigenSolver< concepts::Cmplx >.
Definition at line 642 of file arpackpp.hh.
◆ getRESID()
|
inline |
Returns the RESID vector.
Definition at line 670 of file arpackpp.hh.
◆ info()
|
inlineprotectedvirtual |
Returns information in an output stream.
Reimplemented from eigensolver::EigenSolver< concepts::Cmplx >.
Definition at line 677 of file arpackpp.hh.
◆ iterations() [1/2]
|
inlinevirtual |
Returns the actual number of Arnoldi iterations.
Definition at line 660 of file arpackpp.hh.
◆ iterations() [2/2]
|
pure virtualinherited |
Returns the number of iterations.
Implemented in eigensolver::ArPackppGen< typename eigensolver::OperatorType< F, concepts::Real >::type, F, concepts::Real >, eigensolver::ArPackppStd< typename eigensolver::OperatorType< Cmplx, Real >::type >, and eigensolver::ArPackppStd< F >.
Member Data Documentation
◆ aow_
|
private |
Wrapper.
Definition at line 727 of file arpackpp.hh.
◆ arpackSolver_
|
private |
Arpack solver.
Definition at line 731 of file arpackpp.hh.
◆ computed_
|
private |
Whether or not eigenpairs have already been computed.
Definition at line 740 of file arpackpp.hh.
◆ convEigenvalues_
|
private |
Number of converged eigenpairs.
Definition at line 737 of file arpackpp.hh.
◆ eigenValues_
|
private |
References into storage for eigenvalues.
Definition at line 743 of file arpackpp.hh.
◆ eigenVectors_
|
private |
References into storage for eigenvectors.
Definition at line 746 of file arpackpp.hh.
◆ iter_
|
private |
Number of Arnoldi iterations.
Definition at line 734 of file arpackpp.hh.
◆ modus_
|
private |
Mode of the Arpack solver.
Definition at line 721 of file arpackpp.hh.
◆ whichEV_
|
private |
Identifier indicating which eigenvalues should be calculated.
Definition at line 718 of file arpackpp.hh.
The documentation for this class was generated from the following file:
- eigensolver/arpackpp.hh