eigensolver::DirPowIt< F, G > Class Template Referenceabstract
Eigenvalue solver using the direct power iteration method. More...
#include <DirPowIt.hh>
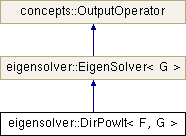
Public Member Functions | |
virtual uint | converged () const |
Returns the number of converged eigenpairs (not implemented) More... | |
virtual uint | converged () const=0 |
Returns the number of converged eigen pairs. More... | |
DirPowIt (concepts::Operator< F > &A, const int nev=3, const int m=3, const int k=0, const int maxiter=300, concepts::Array< concepts::Vector< G > * > *start=0, const Real tol=0) | |
Constructor. More... | |
virtual const concepts::Array< concepts::Vector< G > * > & | getEF () |
Returns an array with the eigenfunctions. More... | |
virtual const concepts::Array< G > & | getEV () |
Returns an array with the eigenvalues. More... | |
virtual uint | iterations () const |
Returns the number of iterations. More... | |
virtual uint | iterations () const=0 |
Returns the number of iterations. More... | |
virtual | ~DirPowIt () |
Destructor. More... | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
void | compute_ () |
Compute eigenpairs. More... | |
void | sort_ (concepts::Array< Real > &) |
Sorting an array using bubble sort and returning the permutation: More... | |
Private Attributes | |
concepts::Operator< F > & | A_ |
Operator A. More... | |
bool | computed_ |
Are the eigenpairs already computed ? More... | |
concepts::Array< G > | eigenvalues_ |
Storage space for eigenvalues. More... | |
concepts::Array< concepts::Vector< G > * > | eigenvectors_ |
Storage space for eigenvectors. More... | |
int | iter_ |
Number of iterations. More... | |
int | k_ |
Number of highest eigenvalues to be cut off: More... | |
int | m_ |
Size of initial matrix V;. More... | |
int | maxiter_ |
Maximum number of iterations allowed. More... | |
int | nev_ |
Number of eigenpairs to be computed. More... | |
concepts::Array< uint > | permutation_ |
concepts::Array< concepts::Vector< G > * > | temp_eigenvectors_ |
Temporary storage space for eigenvectors. More... | |
Real | tol_ |
Convergence tolerance for the eigenpairs. More... | |
Detailed Description
template<typename F, typename G>
class eigensolver::DirPowIt< F, G >
Eigenvalue solver using the direct power iteration method.
Definition at line 31 of file DirPowIt.hh.
Constructor & Destructor Documentation
◆ DirPowIt()
eigensolver::DirPowIt< F, G >::DirPowIt | ( | concepts::Operator< F > & | A, |
const int | nev = 3 , |
||
const int | m = 3 , |
||
const int | k = 0 , |
||
const int | maxiter = 300 , |
||
concepts::Array< concepts::Vector< G > * > * | start = 0 , |
||
const Real | tol = 0 |
||
) |
Constructor.
- Parameters
-
A Operator A of which we want the eigenpairs nev Number of eigenpairs to be computed m Number of columns of the iteration matrix V (must be equal to the number of columns of start) k It is recommended to set k = 0; k was intended to cut out the largest k from the nev eigenvalues; but to really implement such a feature one would have to do it in a more sophisticated way. Presently if k != 0 sometimes the smallest and sometimes the largest k from the nev eigenvalues are cut out. Nevertehless it seems to work for k >= 0.5*nev. maxiter Maximum number of iterations allowed start Initial n x m array (estimate for the eigenvectors) for iteration (n is the dimension of A and m must be bigger than nev) tol Convergence tolerance for the eigenpairs. The default value is the machine precision for double numbers.
The routine implements the following algorithm:
n = size(A,1); V = zeros(n,m); / start d = zeros(k,1); for i = 1 : maxiter V = A*V; [Q,R] = qr(V,0); T = Q'*A*Q; [S,D] = eig(T); [l,perm] = sort(-abs(diag(D))); V_new = Q*S(:,perm); if (norm(d+l(1:k)) < tol), break; end; V = V_new; d = -l(1:k); iter = iter + 1; end; V = V_new(:,1:k); l = -l(1:k);
◆ ~DirPowIt()
|
virtual |
Destructor.
Member Function Documentation
◆ compute_()
|
private |
Compute eigenpairs.
◆ converged() [1/2]
|
inlinevirtual |
Returns the number of converged eigenpairs (not implemented)
Definition at line 85 of file DirPowIt.hh.
◆ converged() [2/2]
|
pure virtualinherited |
Returns the number of converged eigen pairs.
◆ getEF()
|
virtual |
Returns an array with the eigenfunctions.
Implements eigensolver::EigenSolver< G >.
◆ getEV()
|
virtual |
Returns an array with the eigenvalues.
Implements eigensolver::EigenSolver< G >.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from eigensolver::EigenSolver< G >.
◆ iterations() [1/2]
|
inlinevirtual |
Returns the number of iterations.
Definition at line 83 of file DirPowIt.hh.
◆ iterations() [2/2]
|
pure virtualinherited |
Returns the number of iterations.
◆ sort_()
|
private |
Sorting an array using bubble sort and returning the permutation:
Member Data Documentation
◆ A_
|
private |
Operator A.
Definition at line 90 of file DirPowIt.hh.
◆ computed_
|
private |
Are the eigenpairs already computed ?
Definition at line 113 of file DirPowIt.hh.
◆ eigenvalues_
|
private |
Storage space for eigenvalues.
Definition at line 104 of file DirPowIt.hh.
◆ eigenvectors_
|
private |
Storage space for eigenvectors.
Definition at line 106 of file DirPowIt.hh.
◆ iter_
|
private |
Number of iterations.
Definition at line 98 of file DirPowIt.hh.
◆ k_
|
private |
Number of highest eigenvalues to be cut off:
Definition at line 96 of file DirPowIt.hh.
◆ m_
|
private |
Size of initial matrix V;.
Definition at line 94 of file DirPowIt.hh.
◆ maxiter_
|
private |
Maximum number of iterations allowed.
Definition at line 100 of file DirPowIt.hh.
◆ nev_
|
private |
Number of eigenpairs to be computed.
Definition at line 92 of file DirPowIt.hh.
◆ permutation_
|
private |
Definition at line 111 of file DirPowIt.hh.
◆ temp_eigenvectors_
|
private |
Temporary storage space for eigenvectors.
Definition at line 108 of file DirPowIt.hh.
◆ tol_
|
private |
Convergence tolerance for the eigenpairs.
Definition at line 102 of file DirPowIt.hh.
The documentation for this class was generated from the following file:
- eigensolver/DirPowIt.hh