hp2D::Maxwell2D_E Class Referenceabstract
Class for calculating Eddy current problem with Maxwell modell in e-formulation. More...
#include <Maxwell2D_E.hh>
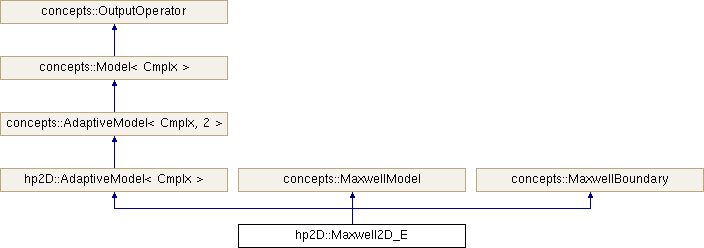
Public Types | |
enum | boundaryType { PMC = 0, PEC = 1, MAX_TYPE } |
Boundary type. More... | |
enum | solverType { SUPERLU = 0, BICGSTAB } |
Type of the solver. More... | |
typedef Cmplx | type |
Public Member Functions | |
boundaryType & | bType () |
const boundaryType | bType () const |
Returns boundary type. More... | |
const std::string | bTypeStr () const |
Returns name of boundary type as string. More... | |
virtual Real | dissipation () |
Return dissipation power loss. More... | |
virtual Real | magnEnergy () |
Return magnetic energy. More... | |
Maxwell2D_E (concepts::EddyGeometry2D &geom, enum boundaryType bType=PEC, enum solverType type=SUPERLU, bool diagPrecond=true, bool afterIter=false, const Real eps=EPS0, const Real omega=OMEGA50, const Real mu=MU0, const uint geomRefAttrib=100) | |
Maxwell2D_E (concepts::EddyGeometry2D &geom, InputMaxwell2D_E &input, const uint geomRefAttrib=100) | |
void | rebuildMesh (concepts::InputAdaptiveModels &input) |
void | rebuildMesh (const uint l=0, const uint p=1, const uint g=0, const uint subdiv=X|Y) |
Rebuilds only the mesh and sets the polynomial degrees. More... | |
const Vector< Cmplx > * | solution () |
Returns solution vector. More... | |
virtual hp2D::hpAdaptiveSpaceHCurl & | space () const |
Returns the space. More... | |
virtual Space< Real > & | space () const=0 |
Returns the space. More... | |
virtual | ~Maxwell2D_E () |
Protected Types | |
enum | subdivTypes |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
virtual concepts::Mesh & | mesh_ () |
Mesh. More... | |
virtual const std::string | mshAbbr_ () |
Mesh abbreviation string. More... | |
virtual hpFull & | prebuild_ () |
Space Prebuilder. More... | |
virtual void | rebuildMesh_ (const uint l=0, const uint p=1, const uint g=0, const uint subdiv=X|Y) |
Rebuilds only the mesh and sets the polynomial degrees. More... | |
Protected Attributes | |
const uint | geomRefAttr_ |
Attribute of vertices or edges for geometric refinement. More... | |
const std::string | problemName_ |
Name of the problem. More... | |
std::unique_ptr< Vector< Cmplx > > | sol_ |
Solution vector. More... | |
Private Member Functions | |
void | constructor_ () |
Private constructor. More... | |
void | identityMatrix_ () |
Calculate identity matrix. More... | |
void | linearform_ () |
Calculate the load vector, assumes sigma = 0 inside the coil. More... | |
void | matrices_ () |
Building the matrices. More... | |
void | rotrotMatrix_ () |
Calculate stiffness matrix. More... | |
virtual void | solve_ () |
Method for solving, throws exception when it wasn't successfull. More... | |
Private Attributes | |
std::unique_ptr< concepts::SparseMatrix< Real > > | A_ |
Mass matrix. More... | |
bool | afterIter_ |
Nachiteration. More... | |
std::unique_ptr< concepts::BoundaryConditions > | bc_ |
Boundary conditions. More... | |
enum boundaryType | bType_ |
Type of boundary condition. More... | |
bool | diagPrecond_ |
Using diagonal preconditioning. More... | |
std::unique_ptr< Real > | dissipation_ |
Dissipation power loss. More... | |
const Real | eps_ |
Dielectricity constant. More... | |
concepts::EddyGeometry2D & | geom_ |
Mesh and material constants (sigma, j0) More... | |
std::unique_ptr< concepts::SparseMatrix< Real > > | M_eddy_ |
std::unique_ptr< concepts::SparseMatrix< Real > > | M_wave_ |
std::unique_ptr< Real > | magnEnergy_ |
Magnetic energy. More... | |
double | matrixtime_ |
const Real | mu_ |
Permeability constant. More... | |
const Real | omega_ |
Angular frequency. More... | |
std::unique_ptr< concepts::Vector< Cmplx > > | residual_ |
Residual of solving the linear system. More... | |
std::unique_ptr< Real > | residualNorm_ |
Euclidian norm of the residual of solving the linear system. More... | |
std::unique_ptr< concepts::Vector< Cmplx > > | rhs_ |
std::unique_ptr< concepts::SparseMatrix< Cmplx > > | S_ |
Stiffness and System matrix. More... | |
double | solvetime_ |
Time to solve the system, to build the matrices, to rebuild the space. More... | |
double | spacetime_ |
std::unique_ptr< hp2D::hpAdaptiveSpaceHCurl > | spc_ |
Space. More... | |
bool | statusAfterIter_ |
enum solverType | type_ |
Solver type. More... | |
Friends | |
class | concepts::ModelControl< Maxwell2D_E > |
Detailed Description
Class for calculating Eddy current problem with Maxwell modell in e-formulation.
Definition at line 32 of file Maxwell2D_E.hh.
Member Typedef Documentation
◆ type
|
inherited |
Member Enumeration Documentation
◆ boundaryType
|
inherited |
Boundary type.
Either perfect magnetic conductor (PMC) or perfect electric conductor (PEC). Wether it's dirichlet or neumann boundary is dependent from the formulation.
Enumerator | |
---|---|
PMC | |
PEC | |
MAX_TYPE |
Definition at line 27 of file maxwell.hh.
◆ solverType
◆ subdivTypes
|
protectedinherited |
Definition at line 83 of file adaptiveModels.hh.
Constructor & Destructor Documentation
◆ Maxwell2D_E() [1/2]
hp2D::Maxwell2D_E::Maxwell2D_E | ( | concepts::EddyGeometry2D & | geom, |
enum boundaryType | bType = PEC , |
||
enum solverType | type = SUPERLU , |
||
bool | diagPrecond = true , |
||
bool | afterIter = false , |
||
const Real | eps = EPS0 , |
||
const Real | omega = OMEGA50 , |
||
const Real | mu = MU0 , |
||
const uint | geomRefAttrib = 100 |
||
) |
◆ Maxwell2D_E() [2/2]
hp2D::Maxwell2D_E::Maxwell2D_E | ( | concepts::EddyGeometry2D & | geom, |
InputMaxwell2D_E & | input, | ||
const uint | geomRefAttrib = 100 |
||
) |
◆ ~Maxwell2D_E()
|
inlinevirtual |
Definition at line 46 of file Maxwell2D_E.hh.
Member Function Documentation
◆ bType() [1/2]
|
inlineinherited |
Definition at line 34 of file maxwell.hh.
◆ bType() [2/2]
|
inlineinherited |
Returns boundary type.
Definition at line 33 of file maxwell.hh.
◆ bTypeStr()
|
inlineinherited |
Returns name of boundary type as string.
Definition at line 36 of file maxwell.hh.
◆ constructor_()
|
private |
Private constructor.
◆ dissipation()
|
virtual |
Return dissipation power loss.
Implements concepts::MaxwellModel.
◆ identityMatrix_()
|
private |
Calculate identity matrix.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::Model< Cmplx >.
◆ linearform_()
|
private |
Calculate the load vector, assumes sigma = 0 inside the coil.
◆ magnEnergy()
|
virtual |
Return magnetic energy.
Implements concepts::MaxwellModel.
◆ matrices_()
|
private |
Building the matrices.
◆ mesh_()
|
inlineprotectedvirtualinherited |
◆ mshAbbr_()
|
inlineprotectedvirtual |
Mesh abbreviation string.
Implements concepts::Model< Cmplx >.
Definition at line 58 of file Maxwell2D_E.hh.
◆ prebuild_()
|
inlineprotectedvirtual |
Space Prebuilder.
Implements hp2D::AdaptiveModel< Cmplx >.
Definition at line 56 of file Maxwell2D_E.hh.
◆ rebuildMesh() [1/2]
|
inherited |
◆ rebuildMesh() [2/2]
|
inherited |
Rebuilds only the mesh and sets the polynomial degrees.
- Parameters
-
l number of uniform refinements p number of polynomial enlargements g number of geometric refinements subdiv possibility to restrict subdivision strategy for geometric refinement
◆ rebuildMesh_()
|
protectedvirtualinherited |
Rebuilds only the mesh and sets the polynomial degrees.
Implements concepts::AdaptiveModel< Cmplx, 2 >.
◆ rotrotMatrix_()
|
private |
Calculate stiffness matrix.
◆ solution()
|
inlineinherited |
◆ solve_()
|
privatevirtual |
Method for solving, throws exception when it wasn't successfull.
Implements concepts::Model< Cmplx >.
◆ space() [1/2]
|
inlinevirtual |
Returns the space.
Definition at line 48 of file Maxwell2D_E.hh.
◆ space() [2/2]
|
pure virtualinherited |
Returns the space.
Friends And Related Function Documentation
◆ concepts::ModelControl< Maxwell2D_E >
|
friend |
Definition at line 1 of file Maxwell2D_E.hh.
Member Data Documentation
◆ A_
|
private |
Mass matrix.
Definition at line 83 of file Maxwell2D_E.hh.
◆ afterIter_
|
private |
Nachiteration.
Definition at line 75 of file Maxwell2D_E.hh.
◆ bc_
|
private |
Boundary conditions.
Definition at line 68 of file Maxwell2D_E.hh.
◆ bType_
|
privateinherited |
Type of boundary condition.
Definition at line 36 of file maxwell.hh.
◆ diagPrecond_
|
private |
Using diagonal preconditioning.
Definition at line 73 of file Maxwell2D_E.hh.
◆ dissipation_
|
private |
Dissipation power loss.
Definition at line 103 of file Maxwell2D_E.hh.
◆ eps_
|
private |
Dielectricity constant.
Definition at line 87 of file Maxwell2D_E.hh.
◆ geom_
|
private |
Mesh and material constants (sigma, j0)
Definition at line 64 of file Maxwell2D_E.hh.
◆ geomRefAttr_
|
protectedinherited |
Attribute of vertices or edges for geometric refinement.
Definition at line 91 of file adaptiveModels.hh.
◆ M_eddy_
|
private |
Definition at line 83 of file Maxwell2D_E.hh.
◆ M_wave_
|
private |
Definition at line 83 of file Maxwell2D_E.hh.
◆ magnEnergy_
|
private |
Magnetic energy.
Definition at line 105 of file Maxwell2D_E.hh.
◆ matrixtime_
|
private |
Definition at line 107 of file Maxwell2D_E.hh.
◆ mu_
|
private |
Permeability constant.
Definition at line 91 of file Maxwell2D_E.hh.
◆ omega_
|
private |
Angular frequency.
Definition at line 89 of file Maxwell2D_E.hh.
◆ problemName_
|
protectedinherited |
◆ residual_
|
private |
Residual of solving the linear system.
Definition at line 77 of file Maxwell2D_E.hh.
◆ residualNorm_
|
private |
Euclidian norm of the residual of solving the linear system.
Definition at line 79 of file Maxwell2D_E.hh.
◆ rhs_
|
private |
Definition at line 85 of file Maxwell2D_E.hh.
◆ S_
|
private |
Stiffness and System matrix.
Definition at line 81 of file Maxwell2D_E.hh.
◆ sol_
|
protectedinherited |
◆ solvetime_
|
private |
Time to solve the system, to build the matrices, to rebuild the space.
Definition at line 107 of file Maxwell2D_E.hh.
◆ spacetime_
|
private |
Definition at line 107 of file Maxwell2D_E.hh.
◆ spc_
|
private |
Definition at line 66 of file Maxwell2D_E.hh.
◆ statusAfterIter_
|
private |
Definition at line 75 of file Maxwell2D_E.hh.
◆ type_
|
private |
Solver type.
Definition at line 68 of file Maxwell2D_E.hh.
The documentation for this class was generated from the following file:
- models/Maxwell2D_E.hh