concepts::BiCGStab< F, G > Class Template Reference
Solves a symmetric system of linear equations with BiConjugate Gradient Stabilized (BICGSTAB). More...
#include <bicgstab.hh>
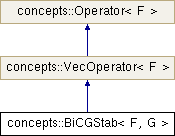
Public Types | |
typedef Cmplxtype< F >::type | c_type |
Real type of data type. More... | |
typedef Realtype< F >::type | r_type |
Real type of data type. More... | |
typedef F | type |
Type of data, e.g. matrix entries. More... | |
Public Member Functions | |
BiCGStab (Operator< F > &A, Operator< G > &Minv, Real maxeps, int maxit=0, bool relres=0, bool throwing=true, Operator< G > *M=0) | |
Constructor. More... | |
BiCGStab (Operator< F > &A, Real maxeps, int maxit=0, uint relres=false, bool throwing=true) | |
Constructor. More... | |
virtual const uint | dimX () const |
Returns the size of the image space of the operator (number of rows of the corresponding matrix) More... | |
virtual const uint | dimY () const |
Returns the size of the source space of the operator (number of columns of the corresponding matrix) More... | |
Real | epsilon () const |
Returns the residual. More... | |
uint | iterations () const |
Returns the number of iterations. More... | |
void | operator() () |
Application method without second argument. Used for parallel solvers. More... | |
virtual void | operator() (const Function< c_type > &fncY, Function< c_type > &fncX) |
Application operator for complex function fncY . More... | |
virtual void | operator() (const Function< r_type > &fncY, Function< F > &fncX) |
Application operator for real function fncY . More... | |
void | operator() (const Matrix< c_type > &mX, Matrix< c_type > &mY) |
Application method to complex matrices. Calls apply_() More... | |
void | operator() (const Matrix< r_type > &mX, Matrix< F > &mY) |
Application method to real matrices. Calls function apply() More... | |
virtual void | operator() (const Vector< c_type > &fncY, Vector< c_type > &fncX) |
Application operator for complex function fncY . More... | |
virtual void | operator() (const Vector< r_type > &fncY, Vector< F > &fncX) |
Application operator for real vector fncY . More... | |
virtual void | show_messages () |
bool | stagnated () const |
Return true, if solver stagnated. More... | |
Protected Member Functions | |
std::ostream & | info (std::ostream &os) const |
Protected Attributes | |
uint | dimX_ |
Dimension of image space and the source space. More... | |
uint | dimY_ |
Private Member Functions | |
virtual void | apply_ () |
Intrinsic application method without argument. More... | |
virtual void | apply_ (const Vector< F > &fncY, Vector< F > &fncX) |
Intrinsic application method, i.e. More... | |
bool | converged_ (const Vector< F > &fncY, Vector< F > &fncX, const Vector< F > &x, const uint &it, Real &eps, const Real &maxeps, const Real &l2Y) |
Calculated current residual and return true if its smaller then maxeps . More... | |
template<class I > | |
bool | scalarTooSmall_ (I &scalar, const std::string name, const uint &it, const Real &eps, const Real &maxeps) const |
Checks if the scalar is zero or NaN and throws an exception in this case. More... | |
bool | stagnated_ (const Vector< F > &x, const Vector< F > &s_p, F &alpha_omega) |
Private Attributes | |
Operator< F > & | A_ |
Operator which is solved. More... | |
Real | eps_ |
Current residual. More... | |
concepts::Sequence< Real > | epsVec_ |
All residual. More... | |
uint | it_ |
Number of iterations. More... | |
Operator< G > * | M_ |
Operator which inverse is Minv, for control of residual. More... | |
Real | maxeps_ |
Convergence criterion. More... | |
uint | maxit_ |
Maximal number of iterations until abortion. More... | |
bool | relres_ |
false: absolute residual, true: relative residual More... | |
bool | stag_ |
true: solver stagnated More... | |
bool | throwing_ |
false: best solution is given, when non converging true: exception is thrown, when non converging More... | |
Operator< G > * | W_ |
Optional preconditioner. More... | |
Detailed Description
template<class F, class G = F>
class concepts::BiCGStab< F, G >
Solves a symmetric system of linear equations with BiConjugate Gradient Stabilized (BICGSTAB).
Constructing an object of this class does not solve the given system. Use the application operator to solve the system. If you want to specify a starting vector for the cg iterations, set fncX
before calling the application operator to this starting value. fncX
also holds the result after the solve.
The application operator throws NoConvergence
if the desired residual maxeps
is not reached within the given number of iterations maxit
.
Definition at line 34 of file bicgstab.hh.
Member Typedef Documentation
◆ c_type
|
inherited |
Real type of data type.
Definition at line 120 of file compositions.hh.
◆ r_type
|
inherited |
Real type of data type.
Definition at line 118 of file compositions.hh.
◆ type
|
inherited |
Type of data, e.g. matrix entries.
Definition at line 45 of file compositions.hh.
Constructor & Destructor Documentation
◆ BiCGStab() [1/2]
|
inline |
Constructor.
- Parameters
-
A Matrix maxeps Maximal residual maxit Maximal number of iterations relres Relative residual throwing In the case of non convergence an exception is thrown and the best solution is not given back.
Definition at line 44 of file bicgstab.hh.
◆ BiCGStab() [2/2]
|
inline |
Constructor.
- Parameters
-
A Matrix Minv Preconditioner for A, ie. Minv should approximate and it has to be symmetric positive definite
maxeps Maximal residual maxit Maximal number of iterations relres Relative residual throwing In the case of non convergence an exception is thrown and the best solution is not given back. M Operator which inverse is Minv, for control of residual
Definition at line 61 of file bicgstab.hh.
Member Function Documentation
◆ apply_() [1/2]
|
privatevirtual |
Intrinsic application method without argument.
Implements concepts::VecOperator< F >.
◆ apply_() [2/2]
|
privatevirtual |
Intrinsic application method, i.e.
real Operator and real Vector or complex Operator and real Vector.
Implements concepts::VecOperator< F >.
◆ converged_()
|
private |
Calculated current residual and return true if its smaller then maxeps
.
◆ dimX()
|
inlinevirtualinherited |
Returns the size of the image space of the operator (number of rows of the corresponding matrix)
Definition at line 93 of file compositions.hh.
◆ dimY()
|
inlinevirtualinherited |
Returns the size of the source space of the operator (number of columns of the corresponding matrix)
Definition at line 98 of file compositions.hh.
◆ epsilon()
|
inline |
Returns the residual.
Calling this method makes only sence after a linear system has been solved.
Definition at line 78 of file bicgstab.hh.
◆ info()
|
protectedvirtual |
Reimplemented from concepts::VecOperator< F >.
◆ iterations()
|
inline |
Returns the number of iterations.
Calling this method makes only sense after a linear system has been solved.
Definition at line 73 of file bicgstab.hh.
◆ operator()() [1/7]
|
virtualinherited |
Application method without second argument. Used for parallel solvers.
Reimplemented from concepts::Operator< F >.
◆ operator()() [2/7]
|
virtualinherited |
Application operator for complex function fncY
.
Computes fncX
= A(fncY
) where A is this operator. fncX
becomes complex.
In derived classes its enough to implement the operator() for complex Operator's. If a real counterpart is not implemented, the function fncY
is splitted into real and imaginary part and the application operator for real functions is called for each. Then the result is combined.
If in a derived class the operator() for complex Operator's is not implemented, a exception is thrown from here.
Reimplemented from concepts::Operator< F >.
◆ operator()() [3/7]
|
virtualinherited |
Application operator for real function fncY
.
Computes fncX
= A(fncY
) where A is this operator.
fncX
becomes the type of the operator, for real data it becomes real, for complex data it becomes complex.
In derived classes its enough to implement the operator() for real Operator's. If a complex counterpart is not implemented, the function fncY
is transformed to a complex function and then the application operator for complex functions is called.
If in a derived class the operator() for real Operator's is not implemented, a exception is thrown from here.
Reimplemented from concepts::Operator< F >.
◆ operator()() [4/7]
|
inherited |
Application method to complex matrices. Calls apply_()
◆ operator()() [5/7]
|
inherited |
Application method to real matrices. Calls function apply()
◆ operator()() [6/7]
|
virtualinherited |
Application operator for complex function fncY
.
Computes fncX
= A(fncY
) where A is this operator. fncX
becomes complex.
In derived classes its enough to implement the operator() for complex Operator's. If a real counterpart is not implemented, the vector fncY
is splitted into real and imaginary part and the application operator for real vectors is called for each. Then the result is combined
If in a derived class the operator() for complex Operator's i not implemented, a exception is thrown from here.
◆ operator()() [7/7]
|
virtualinherited |
Application operator for real vector fncY
.
Computes fncX
= A(fncY
) where A is this operator.
Type of fncX
becomes that of the operator, for real data it becomes real, for complex data it becomes complex.
In derived classes its enough to implement the operator() for real Operator's. If a complex counterpart is not implemented, the vector fncY
is transformed to a complex vector and then the application for complex vectors is called.
If in a derived class the operator() for real Operator's is not implemented, a exception is thrown from here.
◆ scalarTooSmall_()
|
private |
Checks if the scalar is zero or NaN and throws an exception in this case.
◆ show_messages()
|
inlinevirtualinherited |
Reimplemented in concepts::Newton< F >, concepts::MumpsOverlap< F >, and concepts::Mumps< F >.
Definition at line 100 of file compositions.hh.
◆ stagnated()
|
inline |
Return true, if solver stagnated.
Definition at line 80 of file bicgstab.hh.
◆ stagnated_()
|
private |
Member Data Documentation
◆ A_
|
private |
Operator which is solved.
Definition at line 87 of file bicgstab.hh.
◆ dimX_
|
protectedinherited |
Dimension of image space and the source space.
Definition at line 104 of file compositions.hh.
◆ dimY_
|
protectedinherited |
Definition at line 104 of file compositions.hh.
◆ eps_
|
private |
Current residual.
Definition at line 96 of file bicgstab.hh.
◆ epsVec_
|
private |
All residual.
Definition at line 98 of file bicgstab.hh.
◆ it_
|
private |
Number of iterations.
Definition at line 100 of file bicgstab.hh.
◆ M_
|
private |
Operator which inverse is Minv, for control of residual.
Definition at line 89 of file bicgstab.hh.
◆ maxeps_
|
private |
Convergence criterion.
Definition at line 92 of file bicgstab.hh.
◆ maxit_
|
private |
Maximal number of iterations until abortion.
Definition at line 94 of file bicgstab.hh.
◆ relres_
|
private |
false: absolute residual, true: relative residual
Definition at line 102 of file bicgstab.hh.
◆ stag_
|
private |
true: solver stagnated
Definition at line 108 of file bicgstab.hh.
◆ throwing_
|
private |
false: best solution is given, when non converging true: exception is thrown, when non converging
Definition at line 106 of file bicgstab.hh.
◆ W_
|
private |
Optional preconditioner.
Definition at line 85 of file bicgstab.hh.
The documentation for this class was generated from the following file:
- operator/bicgstab.hh