concepts::Hexahedron3d Class Reference
A 3D cell: hexahedron. More...
#include <cell3D.hh>
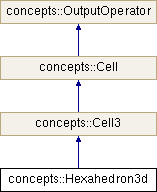
Classes | |
struct | Index |
Subclass of Hexahedron3d representing its index. More... | |
Public Types | |
typedef uint | index_type |
Public Member Functions | |
Real3d | center () const |
Returns the center of the cell. More... | |
Real3d | chi (Real xi, Real eta, Real zeta) const |
The element map. More... | |
virtual Hexahedron3d * | child (uint i) |
Returns a pointer to the ith child. More... | |
virtual const Hexahedron3d * | child (uint i) const |
Returns a pointer to the ith child. More... | |
Hexahedron & | connector () const |
Returns the connector. More... | |
virtual Real3d | elemMap (const Real coord_local) const |
Element map from point local coordinates in 1D. More... | |
virtual Real3d | elemMap (const Real2d &coord_local) const |
Element map from point local coordinates in 2D. More... | |
Real3d | elemMap (const Real3d &coord_local) const |
Element map from point local coordinates in 3D. More... | |
MappingQuad3d * | faceMap (uint face) const |
Returns the mapping of a face. More... | |
const Hex3dSubdivision * | getStrategy () const |
Returns the subdivision strategy of this hexahedron. More... | |
bool | hasChildren () const |
Returns true if there is a least one child. More... | |
MapReal3d | hessian (const uint i, const concepts::Real3d &p) const |
MapReal3d | hessian (const uint i, const Real xi, const Real eta, const Real zeta) const |
Hexahedron3d (Hexahedron &cntr, const MappingHexahedron3d &map) | |
Constructor. More... | |
MapReal3d | jacobian (const concepts::Real3d &p) const |
MapReal3d | jacobian (const Real xi, const Real eta, const Real zeta) const |
Computes the Jacobian for xi , eta , zeta ![]() | |
Real | jacobianDeterminant (const Real xi, const Real eta, const Real zeta) const |
Returns the determinant of the Jacobian for xi , eta , zeta ![]() | |
MapReal3d | jacobianInverse (const Real xi, const Real eta, const Real zeta) const |
Returns the inverse of the Jacobian for xi , eta , zeta ![]() | |
const Level< 3 > & | level () const |
Returns the level of the cell. More... | |
const MappingHexahedron3d * | map () const |
Returns the element map. More... | |
void | setStrategy (const Hex3dSubdivision *strategy=0) throw (StrategyChange) |
Sets the subdivision strategy of this hexahedron. More... | |
Real3d | vertex (uint i) const |
Returns the coordinates of the ith vertex. More... | |
virtual | ~Hexahedron3d () |
Static Public Attributes | |
static uint | MAX_LEVEL |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
Hexahedron3d (Hexahedron &cntr, MappingHexahedron3d *map, const Index &idx) | |
Private constructor. More... | |
Private Attributes | |
Hexahedron3d * | chld_ |
Pointer to the first child. More... | |
Hexahedron & | cntr_ |
Reference to the hexahedron connector (topology) More... | |
Index | idx_ |
Index of this element. More... | |
Hexahedron3d * | lnk_ |
Pointer to a sibling. More... | |
MappingHexahedron3d * | map_ |
Pointer to the element map. More... | |
const Hex3dSubdivision * | subdivStrategy_ |
Subdivision strategy for the hexahedron. More... | |
Friends | |
class | Hex3dSubdiv2x |
class | Hex3dSubdiv2y |
class | Hex3dSubdiv2z |
class | Hex3dSubdiv4x |
class | Hex3dSubdiv4y |
class | Hex3dSubdiv4z |
class | Hex3dSubdiv8 |
class | Hex3dSubdivision |
std::ostream & | operator<< (std::ostream &os, const Hexahedron3d::Index &i) |
Detailed Description
A 3D cell: hexahedron.
This cell contains a reference to the topological information of the hexahedron in cntr_
. If a hexahedron is subdivided, two, four or eight new hexahedra are created. This happens automatically, if a child is requested, depending on the strategy that has been set.
The faces of the hexahedron have the following local coordinates in the element:
- face 0 (bottom): z = 0
- face 1 (front): y = 0
- face 2 (right): x = 1
- face 3 (back): y = 1
- face 4 (left): x = 0
- face 5 (top): z = 1
- Examples
- meshes.cc.
Member Typedef Documentation
◆ index_type
typedef uint concepts::Hexahedron3d::index_type |
Constructor & Destructor Documentation
◆ Hexahedron3d() [1/2]
concepts::Hexahedron3d::Hexahedron3d | ( | Hexahedron & | cntr, |
const MappingHexahedron3d & | map | ||
) |
Constructor.
Takes the connector cntr
and the element map map
and creates a cell.
- Parameters
-
cntr Topological information of the hexadron map Element map of the hexahedron
◆ ~Hexahedron3d()
|
virtual |
◆ Hexahedron3d() [2/2]
|
private |
Private constructor.
Member Function Documentation
◆ center()
|
inlinevirtual |
Returns the center of the cell.
Implements concepts::Cell3.
◆ chi()
The element map.
Maps a point from the unit cube onto the element.
- Returns
- Point in 3D in physical coordinates.
- Parameters
-
xi eta zeta
◆ child() [1/2]
|
virtual |
Returns a pointer to the ith child.
Children are created if they do not already exist.
Implements concepts::Cell3.
◆ child() [2/2]
|
virtual |
Returns a pointer to the ith child.
Children are not created if they do not already exist, instead 0 is returned.
Implements concepts::Cell3.
◆ connector()
|
inlinevirtual |
◆ elemMap() [1/3]
Element map from point local coordinates in 1D.
Reimplemented in concepts::Edge2d, concepts::Edge1d, concepts::Sphere3d, and concepts::SphericalSurface3d.
◆ elemMap() [2/3]
Element map from point local coordinates in 2D.
Reimplemented in concepts::Edge2d, concepts::Edge1d, concepts::Sphere3d, concepts::SphericalSurface3d, concepts::Cell2, concepts::Quad3d, concepts::Triangle3d, concepts::InfiniteRect2d, concepts::Quad2d, and concepts::Triangle2d.
◆ elemMap() [3/3]
Element map from point local coordinates in 3D.
Reimplemented from concepts::Cell.
◆ faceMap()
MappingQuad3d* concepts::Hexahedron3d::faceMap | ( | uint | face | ) | const |
Returns the mapping of a face.
Constructs a cell for a face of this Hexahedron.
◆ getStrategy()
|
inline |
Returns the subdivision strategy of this hexahedron.
If you want to find check against another strategy use
hex.getStrategy() == Hex3dSubdiv4x::instance()
◆ hasChildren()
|
inlineinherited |
◆ hessian() [1/2]
MapReal3d concepts::Hexahedron3d::hessian | ( | const uint | i, |
const concepts::Real3d & | p | ||
) | const |
◆ hessian() [2/2]
MapReal3d concepts::Hexahedron3d::hessian | ( | const uint | i, |
const Real | xi, | ||
const Real | eta, | ||
const Real | zeta | ||
) | const |
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Implements concepts::Cell.
◆ jacobian() [1/2]
MapReal3d concepts::Hexahedron3d::jacobian | ( | const concepts::Real3d & | p | ) | const |
◆ jacobian() [2/2]
Computes the Jacobian for xi
, eta
, zeta
.
The Jacobian of a cell in the initial mesh is computed by the element map. The Jacobian of a subdivided cell is computed as follows. Let be the subdivision map in the reference coordinates (0,1)3 and
,
the element maps of K and K' respectively. Then,
The subdivision map H in reference coordinates is
where ,
,
is the level of the cell with respect to the cell in the initial mesh. The derivative of H is
.
Then, the Jacobian of is
The part (without H) is computed by the element map and the part
is computed in
jacobian()
.
◆ jacobianDeterminant()
◆ jacobianInverse()
◆ level()
|
inline |
◆ map()
|
inline |
◆ setStrategy()
void concepts::Hexahedron3d::setStrategy | ( | const Hex3dSubdivision * | strategy = 0 | ) | |
throw | ( | StrategyChange | |||
) |
Sets the subdivision strategy of this hexahedron.
If the parameter is set to 0 (or if the method is called without parameter) the strategy is set to the default (if not already set). The default subdivision strategy is subdivision into 8 children.
@param strategy Pointer to an instance of a subdivision strategy.
- Exceptions
-
StrategyChange if the change is not allowed (the change is not allowed if there are children present or the topological strategy is set).
◆ vertex()
|
virtual |
Returns the coordinates of the ith vertex.
Implements concepts::Cell3.
Friends And Related Function Documentation
◆ Hex3dSubdiv2x
|
friend |
◆ Hex3dSubdiv2y
|
friend |
◆ Hex3dSubdiv2z
|
friend |
◆ Hex3dSubdiv4x
|
friend |
◆ Hex3dSubdiv4y
|
friend |
◆ Hex3dSubdiv4z
|
friend |
◆ Hex3dSubdiv8
|
friend |
◆ Hex3dSubdivision
|
friend |
◆ operator<<
|
friend |
Member Data Documentation
◆ chld_
|
private |
◆ cntr_
|
private |
◆ idx_
|
private |
◆ lnk_
|
private |
◆ map_
|
private |
◆ MAX_LEVEL
◆ subdivStrategy_
|
private |
Subdivision strategy for the hexahedron.
The documentation for this class was generated from the following file:
- geometry/cell3D.hh