concepts::_Matrix_iterator< _Tp, _Ref, _Ptr > Class Template Reference
STL iterator for matrices. More...
#include <matrixIterator.hh>
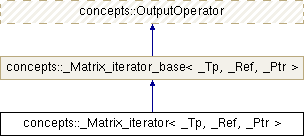
Classes | |
struct | MatrixType |
Non-constant matrix for non-constant iterator. More... | |
struct | MatrixType< _Tp_, const _Tp_ &, const _Tp_ * > |
Constant matrix for constant iterator. More... | |
Public Types | |
typedef _Matrix_iterator_base< _Tp, _Ref, _Ptr > | _Base |
typedef _Matrix_iterator | _Self |
typedef _Matrix_iterator< _Tp, const _Tp &, const _Tp * > | const_iterator |
typedef ptrdiff_t | difference_type |
typedef _Matrix_iterator< _Tp, _Tp &, _Tp * > | iterator |
typedef std::random_access_iterator_tag | iterator_category |
typedef MatrixType< _Tp, _Ref, _Ptr >::type | matrix_type |
typedef _Ptr | pointer |
typedef _Ref | reference |
typedef _Base::return_type | return_type |
typedef size_t | size_type |
typedef _Tp | value_type |
Public Member Functions | |
_Matrix_iterator () | |
Constructor. Iterator stands at the end of any matrix. More... | |
template<class _RefR , class _PtrR > | |
_Matrix_iterator (const _Matrix_iterator< _Tp, _RefR, _PtrR > &__x) | |
Copy constructor. Also from iterator to constant iterator. More... | |
_Matrix_iterator (matrix_type &m, const unsigned int r=0, const unsigned int c=0) | |
Constructor. More... | |
unsigned int | col () const |
Column. More... | |
bool | last () const |
Returns true, if iterator is behind the last entry. More... | |
matrix_type * | matrix () const |
Pointer to the matrix, needed for copy constructor. More... | |
const unsigned int | nofCols () const |
Number of columns. More... | |
const unsigned int | nofRows () const |
Number of rows. More... | |
return_type | operator* () const |
Dereferencation. More... | |
_Self | operator+ (difference_type __n) const |
_Self & | operator++ () |
_Self | operator++ (int) |
_Self & | operator+= (difference_type __n) |
_Self | operator- (difference_type __n) const |
_Self & | operator-- () |
_Self | operator-- (int) |
_Self & | operator-= (difference_type __n) |
pointer | operator-> () const |
Returns a pointer to the value, but only valid for non-constant matrices. More... | |
_Self & | operator= (const iterator &__x) |
Assignment. More... | |
return_type | operator[] (difference_type __n) const |
unsigned int | row () const |
Row. More... | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Protected Attributes | |
unsigned int | col_ |
bool | last_ |
Iterator is behind the last entrance. More... | |
const unsigned int | nofCols_ |
const unsigned int | nofRows_ |
Number of rows and columns. More... | |
unsigned int | row_ |
Current row and column. More... | |
Private Attributes | |
matrix_type * | matrix_ |
Matrix. More... | |
Detailed Description
template<class _Tp, class _Ref, class _Ptr>
class concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >
STL iterator for matrices.
Definition at line 278 of file matrixIterator.hh.
Member Typedef Documentation
◆ _Base
typedef _Matrix_iterator_base<_Tp, _Ref, _Ptr> concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::_Base |
Definition at line 293 of file matrixIterator.hh.
◆ _Self
typedef _Matrix_iterator concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::_Self |
Definition at line 292 of file matrixIterator.hh.
◆ const_iterator
typedef _Matrix_iterator<_Tp, const _Tp&, const _Tp*> concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::const_iterator |
Definition at line 281 of file matrixIterator.hh.
◆ difference_type
typedef ptrdiff_t concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::difference_type |
Definition at line 291 of file matrixIterator.hh.
◆ iterator
typedef _Matrix_iterator<_Tp, _Tp&, _Tp*> concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::iterator |
Definition at line 280 of file matrixIterator.hh.
◆ iterator_category
typedef std::random_access_iterator_tag concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::iterator_category |
Definition at line 286 of file matrixIterator.hh.
◆ matrix_type
typedef MatrixType<_Tp, _Ref, _Ptr>::type concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::matrix_type |
Definition at line 294 of file matrixIterator.hh.
◆ pointer
typedef _Ptr concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::pointer |
Definition at line 288 of file matrixIterator.hh.
◆ reference
typedef _Ref concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::reference |
Definition at line 289 of file matrixIterator.hh.
◆ return_type
typedef _Base::return_type concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::return_type |
Definition at line 295 of file matrixIterator.hh.
◆ size_type
typedef size_t concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::size_type |
Definition at line 290 of file matrixIterator.hh.
◆ value_type
typedef _Tp concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::value_type |
Definition at line 287 of file matrixIterator.hh.
Constructor & Destructor Documentation
◆ _Matrix_iterator() [1/3]
concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::_Matrix_iterator | ( | matrix_type & | m, |
const unsigned int | r = 0 , |
||
const unsigned int | c = 0 |
||
) |
Constructor.
- Parameters
-
m matrix r row c column
◆ _Matrix_iterator() [2/3]
concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >::_Matrix_iterator | ( | ) |
Constructor. Iterator stands at the end of any matrix.
◆ _Matrix_iterator() [3/3]
|
inline |
Copy constructor. Also from iterator to constant iterator.
Definition at line 308 of file matrixIterator.hh.
Member Function Documentation
◆ col()
|
inlineinherited |
Column.
Definition at line 75 of file matrixIterator.hh.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >.
◆ last()
|
inlineinherited |
Returns true, if iterator is behind the last entry.
Definition at line 77 of file matrixIterator.hh.
◆ matrix()
|
inline |
Pointer to the matrix, needed for copy constructor.
Definition at line 328 of file matrixIterator.hh.
◆ nofCols()
|
inlineinherited |
Number of columns.
Definition at line 71 of file matrixIterator.hh.
◆ nofRows()
|
inlineinherited |
Number of rows.
Definition at line 69 of file matrixIterator.hh.
◆ operator*()
|
inline |
Dereferencation.
Definition at line 312 of file matrixIterator.hh.
◆ operator+()
|
inline |
Definition at line 394 of file matrixIterator.hh.
◆ operator++() [1/2]
|
inline |
Definition at line 337 of file matrixIterator.hh.
◆ operator++() [2/2]
|
inline |
Definition at line 347 of file matrixIterator.hh.
◆ operator+=()
|
inline |
Definition at line 375 of file matrixIterator.hh.
◆ operator-()
|
inline |
Definition at line 402 of file matrixIterator.hh.
◆ operator--() [1/2]
|
inline |
Definition at line 353 of file matrixIterator.hh.
◆ operator--() [2/2]
|
inline |
Definition at line 369 of file matrixIterator.hh.
◆ operator-=()
|
inline |
Definition at line 400 of file matrixIterator.hh.
◆ operator->()
|
inline |
Returns a pointer to the value, but only valid for non-constant matrices.
This restriction comes, because the index operator of a constant matrix returns a copy and not a constant reference.
Definition at line 322 of file matrixIterator.hh.
◆ operator=()
|
inline |
Assignment.
Definition at line 331 of file matrixIterator.hh.
◆ operator[]()
|
inline |
Definition at line 407 of file matrixIterator.hh.
◆ row()
|
inlineinherited |
Row.
Definition at line 73 of file matrixIterator.hh.
Member Data Documentation
◆ col_
|
protectedinherited |
Definition at line 93 of file matrixIterator.hh.
◆ last_
|
protectedinherited |
Iterator is behind the last entrance.
Definition at line 95 of file matrixIterator.hh.
◆ matrix_
|
private |
Definition at line 414 of file matrixIterator.hh.
◆ nofCols_
|
protectedinherited |
Definition at line 91 of file matrixIterator.hh.
◆ nofRows_
|
protectedinherited |
Number of rows and columns.
Definition at line 91 of file matrixIterator.hh.
◆ row_
|
protectedinherited |
Current row and column.
Definition at line 93 of file matrixIterator.hh.
The documentation for this class was generated from the following file:
- operator/matrixIterator.hh