concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr > Class Template Reference
Base class for STL like iterator for matrices. More...
#include <matrixIterator.hh>
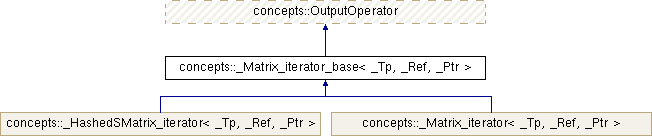
Classes | |
struct | ReturnType |
Return Type reference (_Tp&) for non-constant iterator constant copy (const _Tp) for constant iterator. More... | |
struct | ReturnType< const _Tp_ & > |
Return type for derefencation is constant copy for constant iterator. More... | |
Public Types | |
typedef _Matrix_iterator_base | _Self |
typedef _Matrix_iterator_base< _Tp, const _Tp &, const _Tp * > | const_iterator |
typedef ptrdiff_t | difference_type |
typedef _Matrix_iterator_base< _Tp, _Tp &, _Tp * > | iterator |
typedef _Ptr | pointer |
typedef _Ref | reference |
typedef ReturnType< _Ref >::type | return_type |
typedef size_t | size_type |
typedef _Tp | value_type |
Public Member Functions | |
_Matrix_iterator_base () | |
Constructor. Iterator stands at the end of any matrix. More... | |
template<class _RefR , class _PtrR > | |
_Matrix_iterator_base (const _Matrix_iterator_base< _Tp, _RefR, _PtrR > &__x) | |
Copy constructor. More... | |
_Matrix_iterator_base (const unsigned int nofRows, const unsigned int nofCols, const unsigned int r, const unsigned int c) | |
Constructor. More... | |
unsigned int | col () const |
Column. More... | |
bool | last () const |
Returns true, if iterator is behind the last entry. More... | |
const unsigned int | nofCols () const |
Number of columns. More... | |
const unsigned int | nofRows () const |
Number of rows. More... | |
_Self & | operator= (const iterator &__x) |
Assignment. More... | |
unsigned int | row () const |
Row. More... | |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Protected Attributes | |
unsigned int | col_ |
bool | last_ |
Iterator is behind the last entrance. More... | |
const unsigned int | nofCols_ |
const unsigned int | nofRows_ |
Number of rows and columns. More... | |
unsigned int | row_ |
Current row and column. More... | |
Detailed Description
template<class _Tp, class _Ref, class _Ptr>
class concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >
Base class for STL like iterator for matrices.
Definition at line 28 of file matrixIterator.hh.
Member Typedef Documentation
◆ _Self
typedef _Matrix_iterator_base concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::_Self |
Definition at line 46 of file matrixIterator.hh.
◆ const_iterator
typedef _Matrix_iterator_base<_Tp, const _Tp&, const _Tp*> concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::const_iterator |
Definition at line 31 of file matrixIterator.hh.
◆ difference_type
typedef ptrdiff_t concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::difference_type |
Definition at line 44 of file matrixIterator.hh.
◆ iterator
typedef _Matrix_iterator_base<_Tp, _Tp&, _Tp*> concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::iterator |
Definition at line 30 of file matrixIterator.hh.
◆ pointer
typedef _Ptr concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::pointer |
Definition at line 41 of file matrixIterator.hh.
◆ reference
typedef _Ref concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::reference |
Definition at line 42 of file matrixIterator.hh.
◆ return_type
typedef ReturnType<_Ref>::type concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::return_type |
Definition at line 45 of file matrixIterator.hh.
◆ size_type
typedef size_t concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::size_type |
Definition at line 43 of file matrixIterator.hh.
◆ value_type
typedef _Tp concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::value_type |
Definition at line 40 of file matrixIterator.hh.
Constructor & Destructor Documentation
◆ _Matrix_iterator_base() [1/3]
concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::_Matrix_iterator_base | ( | const unsigned int | nofRows, |
const unsigned int | nofCols, | ||
const unsigned int | r, | ||
const unsigned int | c | ||
) |
Constructor.
- Parameters
-
nofRows number of rows of the matrix nofCols number of columns of the matrix r row c column
◆ _Matrix_iterator_base() [2/3]
concepts::_Matrix_iterator_base< _Tp, _Ref, _Ptr >::_Matrix_iterator_base | ( | ) |
Constructor. Iterator stands at the end of any matrix.
◆ _Matrix_iterator_base() [3/3]
|
inline |
Copy constructor.
Can copy an iterator to an constant one as well.
Definition at line 64 of file matrixIterator.hh.
Member Function Documentation
◆ col()
|
inline |
Column.
Definition at line 75 of file matrixIterator.hh.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
Reimplemented in concepts::_SubMatrix_iterator< F, _Ref, _Ptr >, concepts::_Matrix_iterator< _Tp, _Ref, _Ptr >, and concepts::_HashedSMatrix_iterator< _Tp, _Ref, _Ptr >.
Definition at line 118 of file matrixIterator.hh.
◆ last()
|
inline |
Returns true, if iterator is behind the last entry.
Definition at line 77 of file matrixIterator.hh.
◆ nofCols()
|
inline |
Number of columns.
Definition at line 71 of file matrixIterator.hh.
◆ nofRows()
|
inline |
Number of rows.
Definition at line 69 of file matrixIterator.hh.
◆ operator=()
|
inline |
Assignment.
Definition at line 80 of file matrixIterator.hh.
◆ row()
|
inline |
Row.
Definition at line 73 of file matrixIterator.hh.
Member Data Documentation
◆ col_
|
protected |
Definition at line 93 of file matrixIterator.hh.
◆ last_
|
protected |
Iterator is behind the last entrance.
Definition at line 95 of file matrixIterator.hh.
◆ nofCols_
|
protected |
Definition at line 91 of file matrixIterator.hh.
◆ nofRows_
|
protected |
Number of rows and columns.
Definition at line 91 of file matrixIterator.hh.
◆ row_
|
protected |
Current row and column.
Definition at line 93 of file matrixIterator.hh.
The documentation for this class was generated from the following file:
- operator/matrixIterator.hh