eigensolver::Constrained Class Referenceabstract
Solves a generalized eigenvalue problem subject to linear, homogeneous constraints. More...
#include <constrained.hh>
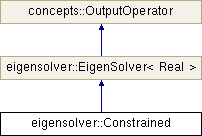
Public Member Functions | |
Constrained (concepts::Operator< Real > &A, concepts::Operator< Real > &M, const concepts::SparseMatrix< Real > &C, SolverFabric< Real > &solver) | |
Constructor. More... | |
virtual uint | converged () const |
virtual uint | converged () const=0 |
Returns the number of converged eigen pairs. More... | |
virtual const concepts::Array< concepts::Vector< Real > * > & | getEF () |
virtual const concepts::Array< Real > & | getEV () |
Returns an array with the eigen values. More... | |
virtual uint | iterations () const |
virtual uint | iterations () const=0 |
Returns the number of iterations. More... | |
virtual | ~Constrained () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Member Functions | |
void | compute_ () |
The real compute routine. More... | |
Private Attributes | |
concepts::Operator< Real > & | A_ |
Stiffness matrix. More... | |
const concepts::SparseMatrix< Real > & | C_ |
Matrix of constraints. ![]() | |
bool | computed_ |
If false, the code will have to compute, otherwise not. More... | |
concepts::Array< Real > | eigenvectors_ |
Storage space for eigenvectors. More... | |
concepts::Array< concepts::Vector< Real > * > | ev_ |
References into storage for eigenvectors. More... | |
concepts::TrivExtendRestrict< Real > * | ext |
uint | it_ |
Statistics. More... | |
uint | k_conv_ |
uint | kmax_ |
Maximal number of eigenvalues which can be computed. More... | |
concepts::Array< Real > | lambda_ |
Storage space for eigenvalues. More... | |
concepts::Operator< Real > & | M_ |
Mass matrix. More... | |
concepts::Permutation< Real > * | Pr |
std::unique_ptr< concepts::Compose< Real > > | PrQgt |
std::unique_ptr< concepts::Permutation< Real > > | Prt |
sparseqr::GivensRotations< Real > * | Qg |
std::unique_ptr< concepts::Compose< Real > > | QgPrt |
std::unique_ptr< concepts::Compose< Real > > | QgPrtext |
sparseqr::GivensRotations< Real > * | Qgt |
concepts::TrivExtendRestrict< Real > * | restr |
std::unique_ptr< concepts::Compose< Real > > | restrPrQgt |
std::unique_ptr< concepts::Compose< Real > > | restrPrQgtA |
std::unique_ptr< concepts::Compose< Real > > | restrPrQgtAQgPrtext |
std::unique_ptr< concepts::Compose< Real > > | restrPrQgtM |
std::unique_ptr< concepts::Compose< Real > > | restrPrQgtMQgPrtext |
SolverFabric< Real > & | solver_ |
Fabric for the eigenvalue solver. More... | |
Detailed Description
Solves a generalized eigenvalue problem subject to linear, homogeneous constraints.
This class solve a generalized eigenvalue problem of the form
subject to the constraints where
and
. This is important for solving eigenvalue problems with essential boundary conditions like
.
For the case , this is described in [1 section 12.6, 2]. For the much more complicated case of
, see [3, 4]. The first case should be sufficient for most applications.
- See also
- [1] Gene H. Golub and Charles F. van Loan, Matrix Computations, The Johns Hopkins University Press, 1983.
[2] Gene H. Golub, Some Modified Matrix Eigenvalue Problems, SIAM Review, 15 (1973), No. 2, 318–334.
[3] Walter Gander, Gene H. Golub and Urs von Matt, A Constrained Eigenvalue Problem, in Numerical Linear Algebra, Digital Signal Processing and Parallel Algorithms, Gene H. Golub and P. Van Dooren (eds.), NATO ASI Series, Vol. F70, 1991, 677–686.
[4] Walter Gander, Gene H. Golub and Urs von Matt, A Constrained Eigenvalue Problem, Linear Algebra and Its Applications, 114/115 (1989), 815–839.
Definition at line 54 of file constrained.hh.
Constructor & Destructor Documentation
◆ Constrained()
|
inline |
Constructor.
- Parameters
-
A Matrix M Matrix C Matrix of constraints solver Fabric for the eigenvalue solver
Definition at line 62 of file constrained.hh.
◆ ~Constrained()
|
virtual |
Member Function Documentation
◆ compute_()
|
private |
◆ converged() [1/2]
|
inlinevirtual |
Definition at line 74 of file constrained.hh.
◆ converged() [2/2]
|
pure virtualinherited |
Returns the number of converged eigen pairs.
Implemented in eigensolver::ArPack< Real >.
◆ getEF()
|
virtual |
Implements eigensolver::EigenSolver< Real >.
◆ getEV()
|
virtual |
Returns an array with the eigen values.
- Deprecated:
- : this interface requires that the returned array must be hold as a member variable of the class.
(use std::auto_pointer or similar)
Implements eigensolver::EigenSolver< Real >.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from eigensolver::EigenSolver< Real >.
◆ iterations() [1/2]
|
inlinevirtual |
Definition at line 73 of file constrained.hh.
◆ iterations() [2/2]
|
pure virtualinherited |
Returns the number of iterations.
Implemented in eigensolver::ArPack< Real >.
Member Data Documentation
◆ A_
|
private |
Stiffness matrix.
Definition at line 89 of file constrained.hh.
◆ C_
|
private |
Matrix of constraints. is enforced.
Definition at line 93 of file constrained.hh.
◆ computed_
|
private |
If false, the code will have to compute, otherwise not.
Definition at line 86 of file constrained.hh.
◆ eigenvectors_
|
private |
Storage space for eigenvectors.
Definition at line 98 of file constrained.hh.
◆ ev_
|
private |
References into storage for eigenvectors.
Definition at line 100 of file constrained.hh.
◆ ext
|
private |
Definition at line 108 of file constrained.hh.
◆ it_
|
private |
Statistics.
Definition at line 114 of file constrained.hh.
◆ k_conv_
|
private |
Definition at line 114 of file constrained.hh.
◆ kmax_
|
private |
Maximal number of eigenvalues which can be computed.
Definition at line 103 of file constrained.hh.
◆ lambda_
|
private |
Storage space for eigenvalues.
Definition at line 96 of file constrained.hh.
◆ M_
|
private |
Mass matrix.
Definition at line 91 of file constrained.hh.
◆ Pr
|
private |
Definition at line 105 of file constrained.hh.
◆ PrQgt
|
private |
Definition at line 109 of file constrained.hh.
◆ Prt
|
private |
Definition at line 106 of file constrained.hh.
◆ Qg
|
private |
Definition at line 107 of file constrained.hh.
◆ QgPrt
|
private |
Definition at line 109 of file constrained.hh.
◆ QgPrtext
|
private |
Definition at line 110 of file constrained.hh.
◆ Qgt
|
private |
Definition at line 107 of file constrained.hh.
◆ restr
|
private |
Definition at line 108 of file constrained.hh.
◆ restrPrQgt
|
private |
Definition at line 109 of file constrained.hh.
◆ restrPrQgtA
|
private |
Definition at line 110 of file constrained.hh.
◆ restrPrQgtAQgPrtext
|
private |
Definition at line 110 of file constrained.hh.
◆ restrPrQgtM
|
private |
Definition at line 110 of file constrained.hh.
◆ restrPrQgtMQgPrtext
|
private |
Definition at line 111 of file constrained.hh.
◆ solver_
|
private |
Fabric for the eigenvalue solver.
Definition at line 79 of file constrained.hh.
The documentation for this class was generated from the following file:
- eigensolver/constrained.hh