geometry::ElementPatch Class Reference
Class holding the informations of a regular patch. More...
#include <patches.hh>
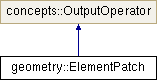
Public Types | |
typedef Sequence< quad_loc >::const_iterator | const_iterator |
typedef Sequence< quad_loc >::iterator | iterator |
enum | PatchType { INNER, NEUMANN_NEUMANN, DIRICHLET_NEUMANN, NEUMANN_DIRICHLET, DIRICHLET_DIRICHLET, NOT_FEATURED_YET } |
Public Member Functions | |
iterator | begin () |
const_iterator | begin () const |
ElementPatch () | |
Default Constructor. More... | |
ElementPatch (const ElementPatch &elmPatch) | |
Copy Constructor. More... | |
iterator | end () |
const_iterator | end () const |
bool | exist (uint elmKey) |
Checks for existing of a key of an element in the Patch. More... | |
Boundary | getBoundaryEdgeType (uint i) const |
Method that returns Boundary of the requested BoundaryEdges in the Patch ( i=0,1 ). More... | |
bool | getPos (uint quadKey, uint &pos) const |
void | insert1st (uint elmKey, uint i) |
quad_loc & | operator[] (uint pos) |
Access operator. More... | |
quad_loc | operator[] (uint pos) const |
Read Operatpor. More... | |
enum PatchType | patchType () const |
Method returns the type of the Element-Patch. More... | |
void | push_back (uint elmKey, uint i) |
Method to insert a (unique) key of an Element. More... | |
uint | size () const |
bool | type () const |
Method to tell the kind of the Elementpatch. More... | |
void | typeUpdateL (concepts::Boundary type) |
void | typeUpdateR (concepts::Boundary type) |
virtual | ~ElementPatch () |
Protected Member Functions | |
std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Attributes | |
Sequence< quad_loc > | elmP_ |
concepts::Boundary | lEdgeType_ |
concepts::Boundary | rEdgeType_ |
Detailed Description
Class holding the informations of a regular patch.
It is a special case of a concepts::Sequence with additional information of the type of the patch. At the moment there are 5 types supported : Inner, Neumann/Neumann, Neumann/Dirichlet, Dirichlet/Dirichlet, Dirichlet/Neumann. This class holds the keys for Elements around a vertex and is sorted and controlled in the controll classes VtxToPatchMaps and VtxtoElmSupportMap.
Definition at line 83 of file patches.hh.
Member Typedef Documentation
◆ const_iterator
Definition at line 99 of file patches.hh.
◆ iterator
Definition at line 98 of file patches.hh.
Member Enumeration Documentation
◆ PatchType
Enumerator | |
---|---|
INNER | |
NEUMANN_NEUMANN | |
DIRICHLET_NEUMANN | |
NEUMANN_DIRICHLET | |
DIRICHLET_DIRICHLET | |
NOT_FEATURED_YET |
Definition at line 89 of file patches.hh.
Constructor & Destructor Documentation
◆ ElementPatch() [1/2]
|
inline |
Default Constructor.
By default the Patch is a Inner Patch, this may be changed with the typeUpdateL or typeUpdateR routines.
Definition at line 106 of file patches.hh.
◆ ElementPatch() [2/2]
|
inline |
Copy Constructor.
Definition at line 114 of file patches.hh.
◆ ~ElementPatch()
|
inlinevirtual |
Definition at line 122 of file patches.hh.
Member Function Documentation
◆ begin() [1/2]
|
inline |
Definition at line 152 of file patches.hh.
◆ begin() [2/2]
|
inline |
Definition at line 156 of file patches.hh.
◆ end() [1/2]
|
inline |
Definition at line 160 of file patches.hh.
◆ end() [2/2]
|
inline |
Definition at line 164 of file patches.hh.
◆ exist()
bool geometry::ElementPatch::exist | ( | uint | elmKey | ) |
Checks for existing of a key of an element in the Patch.
- Parameters
-
Key of the Element.
- Returns
- Returns true if it exists else false.
◆ getBoundaryEdgeType()
Boundary geometry::ElementPatch::getBoundaryEdgeType | ( | uint | i | ) | const |
Method that returns Boundary of the requested BoundaryEdges in the Patch ( i=0,1 ).
- Parameters
-
i No of Edge. i = 0 First (left) boundary edge in the first Quad, i = 1 second (right) boundary edge in the last Quad.
- Returns
- Returns the Boundary of the Edge.
◆ getPos()
bool geometry::ElementPatch::getPos | ( | uint | quadKey, |
uint & | pos | ||
) | const |
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
◆ insert1st()
void geometry::ElementPatch::insert1st | ( | uint | elmKey, |
uint | i | ||
) |
◆ operator[]() [1/2]
|
inline |
Access operator.
- Parameters
-
pos Position for requested element key.
- Returns
- Returns the element-key at the postion.
Definition at line 225 of file patches.hh.
◆ operator[]() [2/2]
|
inline |
Read Operatpor.
- Parameters
-
pos Position for requested element key.
- Returns
- Returns the element-key at the postion.
Definition at line 235 of file patches.hh.
◆ patchType()
enum PatchType geometry::ElementPatch::patchType | ( | ) | const |
Method returns the type of the Element-Patch.
- Returns
- The enum type of the Element-Patch.
◆ push_back()
void geometry::ElementPatch::push_back | ( | uint | elmKey, |
uint | i | ||
) |
Method to insert a (unique) key of an Element.
It is just inserted when not already existing.
- Parameters
-
elmKey Key of the Element.
◆ size()
|
inline |
Definition at line 182 of file patches.hh.
◆ type()
|
inline |
Method to tell the kind of the Elementpatch.
It either returns 0 if the patch is an inner Patch or 1 if the patch is a boundary patch.
- Returns
- a boolish value of the kind of Patch.
Definition at line 193 of file patches.hh.
◆ typeUpdateL()
|
inline |
Definition at line 204 of file patches.hh.
◆ typeUpdateR()
|
inline |
Definition at line 208 of file patches.hh.
Member Data Documentation
◆ elmP_
Definition at line 246 of file patches.hh.
◆ lEdgeType_
|
private |
Definition at line 251 of file patches.hh.
◆ rEdgeType_
|
private |
Definition at line 253 of file patches.hh.
The documentation for this class was generated from the following file:
- estimator/patches.hh