concepts::Boundary Class Reference
Class to describe an element of the boundary. More...
#include <boundary.hh>
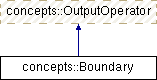
Public Types | |
enum | boundaryTypes { FREE = 0, DIRICHLET, NEUMANN, CONNECT, SPHERESURFACEQUAD, MAX_TYPE } |
The different boundary condition types. More... | |
Public Member Functions | |
Boundary () | |
Default constructor. More... | |
Boundary (const Boundary &bnd) | |
Copy constructor. More... | |
Boundary (const enum boundaryTypes type) | |
Constructor. More... | |
Boundary (const enum boundaryTypes type, const char *frm) | |
Constructor. More... | |
Boundary (const enum boundaryTypes type, const Formula< Real > &frm) | |
Constructor. More... | |
bool | isNull () |
Real | operator() (const Real x, const Real t=0.0) const |
Application operator. More... | |
Real | operator() (const Real2d &x, const Real t=0.0) const |
Application operator. More... | |
Real | operator() (const Real3d &x, const Real t=0.0) const |
Application operator. More... | |
virtual Boundary & | operator= (const Boundary &bnd) |
Assignment operator. More... | |
enum boundaryTypes | type () const |
Returns the type of the boundary condition. More... | |
std::string | typeStr () const |
Return the type of the boundary condition as output string. More... | |
virtual | ~Boundary () |
Protected Member Functions | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
Private Attributes | |
std::unique_ptr< const Formula< Real > > | frm_ |
The function of the boundary condition. More... | |
enum boundaryTypes | type_ |
Type of the boundary. More... | |
Detailed Description
Class to describe an element of the boundary.
A boundary has a type (Neumann, Dirichlet) and a function associated.
Boundary conditions of type Robin are not yet implemented.
It is possible to use this class in the following way:
Boundary(Boundary::NEUMANN, ParsedFormula("(x)"));
since the ParsedFormula
is cloned (ie. it is copied) before the temporary object is destroyed.
- Examples
- elasticity2D_tutorial.cc, howToGetStarted.cc, hpFEM2d-simple.cc, hpFEM2d.cc, hpFEM3d-EV.cc, inhomDirichletBCs.cc, inhomNeumannBCs.cc, linearDG1d.cc, linearFEM1d-simple.cc, linearFEM1d.cc, and parallelizationTutorial.cc.
Definition at line 35 of file boundary.hh.
Member Enumeration Documentation
◆ boundaryTypes
The different boundary condition types.
Enumerator | |
---|---|
FREE | |
DIRICHLET | |
NEUMANN | |
CONNECT | |
SPHERESURFACEQUAD | |
MAX_TYPE |
Definition at line 38 of file boundary.hh.
Constructor & Destructor Documentation
◆ Boundary() [1/5]
concepts::Boundary::Boundary | ( | ) |
Default constructor.
Initializes the type to FREE.
◆ Boundary() [2/5]
concepts::Boundary::Boundary | ( | const enum boundaryTypes | type | ) |
Constructor.
The type of the boundary condition must be one of FREE, DIRICHLET, NEUMANN or CONNECT. In this constructor, no formula can be given. It is always evaluated to 0 by default.
◆ Boundary() [3/5]
concepts::Boundary::Boundary | ( | const enum boundaryTypes | type, |
const Formula< Real > & | frm | ||
) |
◆ Boundary() [4/5]
concepts::Boundary::Boundary | ( | const enum boundaryTypes | type, |
const char * | frm | ||
) |
Constructor.
The type of the boundary condition must be one of FREE, DIRICHLET or NEUMANN. The formula can be given as a string which will be used to create a ParsedFormula
.
◆ Boundary() [5/5]
concepts::Boundary::Boundary | ( | const Boundary & | bnd | ) |
Copy constructor.
◆ ~Boundary()
|
virtual |
Member Function Documentation
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::OutputOperator.
◆ isNull()
|
inline |
Definition at line 82 of file boundary.hh.
◆ operator()() [1/3]
Application operator.
Calculates the value of the boundary function at a specific point.
◆ operator()() [2/3]
Application operator.
Calculates the value of the boundary function at a specific point.
◆ operator()() [3/3]
Application operator.
Calculates the value of the boundary function at a specific point.
◆ operator=()
Assignment operator.
◆ type()
|
inline |
Returns the type of the boundary condition.
Definition at line 71 of file boundary.hh.
◆ typeStr()
std::string concepts::Boundary::typeStr | ( | ) | const |
Return the type of the boundary condition as output string.
Member Data Documentation
◆ frm_
The function of the boundary condition.
Definition at line 106 of file boundary.hh.
◆ type_
|
private |
Type of the boundary.
Definition at line 101 of file boundary.hh.
The documentation for this class was generated from the following file:
- formula/boundary.hh