hp2D::Maxwell2D_H_Base Class Referenceabstract
Base class for calculating Eddy current problem with Maxwell modell in h formulation. More...
#include <Maxwell2D_H.hh>
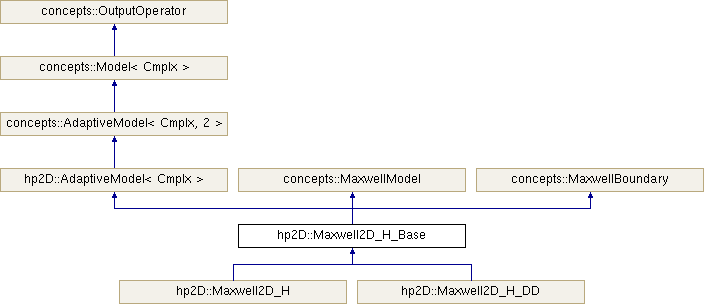
Public Types | |
enum | boundaryType { PMC = 0, PEC = 1, MAX_TYPE } |
Boundary type. More... | |
enum | solverType { SUPERLU = 0, SUPERLU2 = 1, BICGSTAB = 2, BICGSTAB2 = 3, BICGSTABSUPERLU = 4 } |
Type of the solver. More... | |
typedef Cmplx | type |
Public Member Functions | |
boundaryType & | bType () |
const boundaryType | bType () const |
Returns boundary type. More... | |
const std::string | bTypeStr () const |
Returns name of boundary type as string. More... | |
virtual Real | dissipation ()=0 |
Return dissipation power loss. More... | |
virtual Real | magnEnergy ()=0 |
Return magnetic energy. More... | |
Maxwell2D_H_Base (concepts::EddyGeometry2D &geom, enum boundaryType bType=PMC, enum solverType type=SUPERLU, bool diagPrecond=true, bool afterIter=false, const Real eps=EPS0, const Real omega=OMEGA50, const Real mu=MU0, const uint geomRefAttrib=100) | |
Constructor. More... | |
Maxwell2D_H_Base (concepts::EddyGeometry2D &geom, InputMaxwell2D_H &input, const uint geomRefAttrib=100) | |
void | rebuildMesh (concepts::InputAdaptiveModels &input) |
void | rebuildMesh (const uint l=0, const uint p=1, const uint g=0, const uint subdiv=X|Y) |
Rebuilds only the mesh and sets the polynomial degrees. More... | |
const Vector< Cmplx > * | solution () |
Returns solution vector. More... | |
virtual Space< Real > & | space () const=0 |
Returns the space. More... | |
virtual | ~Maxwell2D_H_Base () |
Protected Types | |
enum | subdivTypes |
Protected Member Functions | |
concepts::SparseMatrix< Real > * | identityMatrix_ (concepts::Space< Real > &spc, concepts::SparseMatrix< Cmplx > *S) |
Calculate identity matrix and add's it to system matrix S . More... | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
concepts::SparseMatrix< Cmplx > * | laplaceMatrix_ (concepts::Space< Real > &spc, concepts::SparseMatrix< Cmplx > *S) |
Calculate stiffness matrix and add's it to system matrix S . More... | |
void | linearform_ () |
Calculate the load vector, assumes sigma = 0 inside the coil. More... | |
virtual concepts::Mesh & | mesh_ () |
Mesh. More... | |
virtual const std::string | mshAbbr_ () |
Mesh abbreviation string. More... | |
virtual hpFull & | prebuild_ ()=0 |
Space Prebuilder. More... | |
virtual void | rebuildMesh_ (const uint l=0, const uint p=1, const uint g=0, const uint subdiv=X|Y) |
Rebuilds only the mesh and sets the polynomial degrees. More... | |
virtual void | solve_ ()=0 |
Method for solving. More... | |
Protected Attributes | |
bool | afterIter_ |
Nachiteration. More... | |
std::unique_ptr< concepts::BoundaryConditions > | bc_ |
Boundary conditions. More... | |
bool | diagPrecond_ |
Using diagonal preconditioning. More... | |
std::unique_ptr< Real > | dissipation_ |
Dissipation power loss. More... | |
Real | eps_ |
Dielectricity constant. More... | |
concepts::EddyGeometry2D & | geom_ |
Mesh and material constants (sigma, j0) More... | |
const uint | geomRefAttr_ |
Attribute of vertices or edges for geometric refinement. More... | |
concepts::PiecewiseFormulaFun< Cmplx, Real > | iOmegaEps_plus_Sigma_Inv_ |
Piecewise constant formula. More... | |
uint | iterations_ |
Number of iterations for iterative solver. More... | |
std::unique_ptr< Real > | magnEnergy_ |
Magnetic energy. More... | |
double | matrixtime_ |
const Real | mu_ |
Permeability constant. More... | |
Real | omega_ |
Angular frequency. More... | |
const std::string | problemName_ |
Name of the problem. More... | |
std::unique_ptr< concepts::Vector< Cmplx > > | residual_ |
std::unique_ptr< Real > | residualNorm_ |
Euclidian norm of the residual of solving the linear system. More... | |
std::unique_ptr< concepts::Vector< Cmplx > > | rhs_ |
std::unique_ptr< Vector< Cmplx > > | sol_ |
Solution vector. More... | |
double | solvetime_ |
Time to solve the system, to build the matrices, to rebuild the space. More... | |
double | spacetime_ |
bool | statusAfterIter_ |
enum solverType | type_ |
Solver type. More... | |
Private Member Functions | |
void | constructor_ () |
Private constructor. More... | |
Private Attributes | |
enum boundaryType | bType_ |
Type of boundary condition. More... | |
Friends | |
class | concepts::ModelControl< Maxwell2D_H_Base > |
Detailed Description
Base class for calculating Eddy current problem with Maxwell modell in h formulation.
Definition at line 36 of file Maxwell2D_H.hh.
Member Typedef Documentation
◆ type
|
inherited |
Member Enumeration Documentation
◆ boundaryType
|
inherited |
Boundary type.
Either perfect magnetic conductor (PMC) or perfect electric conductor (PEC). Wether it's dirichlet or neumann boundary is dependent from the formulation.
Enumerator | |
---|---|
PMC | |
PEC | |
MAX_TYPE |
Definition at line 27 of file maxwell.hh.
◆ solverType
Type of the solver.
Enumerator | |
---|---|
SUPERLU | |
SUPERLU2 | |
BICGSTAB | |
BICGSTAB2 | |
BICGSTABSUPERLU |
Definition at line 42 of file Maxwell2D_H.hh.
◆ subdivTypes
|
protectedinherited |
Definition at line 83 of file adaptiveModels.hh.
Constructor & Destructor Documentation
◆ Maxwell2D_H_Base() [1/2]
hp2D::Maxwell2D_H_Base::Maxwell2D_H_Base | ( | concepts::EddyGeometry2D & | geom, |
enum boundaryType | bType = PMC , |
||
enum solverType | type = SUPERLU , |
||
bool | diagPrecond = true , |
||
bool | afterIter = false , |
||
const Real | eps = EPS0 , |
||
const Real | omega = OMEGA50 , |
||
const Real | mu = MU0 , |
||
const uint | geomRefAttrib = 100 |
||
) |
Constructor.
- Parameters
-
geom geometry, conductivity and source currents
bType type of boundary condition type solver type diagPrecond flag for preconditioning with diagonal matrix aterIter flag for after iterations eps dielectricity constant omega angular frequency in 1/s mu permeability constant in Ohm*s/m geomRefAttrib attrib for geometric refinement
◆ Maxwell2D_H_Base() [2/2]
hp2D::Maxwell2D_H_Base::Maxwell2D_H_Base | ( | concepts::EddyGeometry2D & | geom, |
InputMaxwell2D_H & | input, | ||
const uint | geomRefAttrib = 100 |
||
) |
◆ ~Maxwell2D_H_Base()
|
inlinevirtual |
Definition at line 63 of file Maxwell2D_H.hh.
Member Function Documentation
◆ bType() [1/2]
|
inlineinherited |
Definition at line 34 of file maxwell.hh.
◆ bType() [2/2]
|
inlineinherited |
Returns boundary type.
Definition at line 33 of file maxwell.hh.
◆ bTypeStr()
|
inlineinherited |
Returns name of boundary type as string.
Definition at line 36 of file maxwell.hh.
◆ constructor_()
|
private |
Private constructor.
◆ dissipation()
|
pure virtualinherited |
Return dissipation power loss.
Implemented in hp2D::Maxwell2D_H_DD, hp2D::Maxwell2D_H, hp2D::Maxwell2D_E, hp2D::Eddy2D_H, and hp2D::Eddy2D_E.
◆ identityMatrix_()
|
protected |
Calculate identity matrix and add's it to system matrix S
.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::Model< Cmplx >.
Reimplemented in hp2D::Maxwell2D_H_DD, and hp2D::Maxwell2D_H.
◆ laplaceMatrix_()
|
protected |
Calculate stiffness matrix and add's it to system matrix S
.
◆ linearform_()
|
protected |
Calculate the load vector, assumes sigma = 0 inside the coil.
◆ magnEnergy()
|
pure virtualinherited |
Return magnetic energy.
Implemented in hp2D::Maxwell2D_H_DD, hp2D::Maxwell2D_H, hp2D::Maxwell2D_E, hp2D::Eddy2D_H, and hp2D::Eddy2D_E.
◆ mesh_()
|
inlineprotectedvirtualinherited |
◆ mshAbbr_()
|
inlineprotectedvirtual |
Mesh abbreviation string.
Implements concepts::Model< Cmplx >.
Definition at line 67 of file Maxwell2D_H.hh.
◆ prebuild_()
|
protectedpure virtualinherited |
Space Prebuilder.
Implemented in hp2D::Maxwell2D_H_DD, hp2D::Maxwell2D_H, hp2D::Maxwell2D_E, hp2D::Eddy2D_H, and hp2D::Eddy2D_E.
◆ rebuildMesh() [1/2]
|
inherited |
◆ rebuildMesh() [2/2]
|
inherited |
Rebuilds only the mesh and sets the polynomial degrees.
- Parameters
-
l number of uniform refinements p number of polynomial enlargements g number of geometric refinements subdiv possibility to restrict subdivision strategy for geometric refinement
◆ rebuildMesh_()
|
protectedvirtualinherited |
Rebuilds only the mesh and sets the polynomial degrees.
Implements concepts::AdaptiveModel< Cmplx, 2 >.
◆ solution()
|
inlineinherited |
◆ solve_()
|
protectedpure virtualinherited |
Method for solving.
Implemented in hp2D::Maxwell2D_H_DD, hp2D::Maxwell2D_H, hp2D::Maxwell2D_E, hp2D::Eddy2D_H, and hp2D::Eddy2D_E.
◆ space()
|
pure virtualinherited |
Returns the space.
Friends And Related Function Documentation
◆ concepts::ModelControl< Maxwell2D_H_Base >
|
friend |
Definition at line 240 of file Maxwell2D_H.hh.
Member Data Documentation
◆ afterIter_
|
protected |
Nachiteration.
Definition at line 88 of file Maxwell2D_H.hh.
◆ bc_
|
protected |
Boundary conditions.
Definition at line 82 of file Maxwell2D_H.hh.
◆ bType_
|
privateinherited |
Type of boundary condition.
Definition at line 36 of file maxwell.hh.
◆ diagPrecond_
|
protected |
Using diagonal preconditioning.
Definition at line 86 of file Maxwell2D_H.hh.
◆ dissipation_
|
protected |
Dissipation power loss.
Definition at line 103 of file Maxwell2D_H.hh.
◆ eps_
|
protected |
Dielectricity constant.
Definition at line 97 of file Maxwell2D_H.hh.
◆ geom_
|
protected |
Mesh and material constants (sigma, j0)
Definition at line 80 of file Maxwell2D_H.hh.
◆ geomRefAttr_
|
protectedinherited |
Attribute of vertices or edges for geometric refinement.
Definition at line 91 of file adaptiveModels.hh.
◆ iOmegaEps_plus_Sigma_Inv_
|
protected |
◆ iterations_
|
protected |
Number of iterations for iterative solver.
Definition at line 109 of file Maxwell2D_H.hh.
◆ magnEnergy_
|
protected |
Magnetic energy.
Definition at line 105 of file Maxwell2D_H.hh.
◆ matrixtime_
|
protected |
Definition at line 107 of file Maxwell2D_H.hh.
◆ mu_
|
protected |
Permeability constant.
Definition at line 101 of file Maxwell2D_H.hh.
◆ omega_
|
protected |
Angular frequency.
Definition at line 99 of file Maxwell2D_H.hh.
◆ problemName_
|
protectedinherited |
◆ residual_
|
protected |
Definition at line 89 of file Maxwell2D_H.hh.
◆ residualNorm_
|
protected |
Euclidian norm of the residual of solving the linear system.
Definition at line 91 of file Maxwell2D_H.hh.
◆ rhs_
|
protected |
Definition at line 93 of file Maxwell2D_H.hh.
◆ sol_
|
protectedinherited |
◆ solvetime_
|
protected |
Time to solve the system, to build the matrices, to rebuild the space.
Definition at line 107 of file Maxwell2D_H.hh.
◆ spacetime_
|
protected |
Definition at line 107 of file Maxwell2D_H.hh.
◆ statusAfterIter_
|
protected |
Definition at line 88 of file Maxwell2D_H.hh.
◆ type_
|
protected |
Solver type.
Definition at line 82 of file Maxwell2D_H.hh.
The documentation for this class was generated from the following file:
- models/Maxwell2D_H.hh