concepts::Import2dMesh Class Reference
#include <meshImport2D.hh>
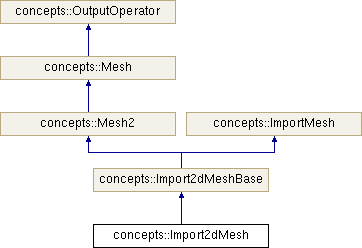
Public Member Functions | |
Import2dMesh (const std::string coord, const std::string elms, const std::string boundary, const uint idxStart=1) | |
Constructor. More... | |
Import2dMesh (const std::string coord, const std::string elms, const uint idxStart=1) | |
Constructor. More... | |
virtual uint | ncell () const |
Returns the number of cells in the mesh. More... | |
virtual Scan2 * | scan () |
Returns a scanner over the cells of the mesh. More... | |
virtual | ~Import2dMesh () |
Protected Member Functions | |
void | attributes_ (const std::string boundary) |
Reads attributes from file boundary . More... | |
template<class T > | |
void | clear_ (std::vector< T * > &field) |
Deletes the content of field of pointers. More... | |
virtual void | createCell_ (const MultiIndex< 2 > &idx) throw (concepts::MissingFeature) |
Creation of geometrical cells with element mapping. More... | |
virtual void | createCell_ (const MultiIndex< 3 > &idx) throw (concepts::MissingFeature) |
Creation of geometrical cells with element mapping. More... | |
virtual void | createCell_ (const MultiIndex< 4 > &idx) throw (concepts::MissingFeature) |
virtual void | createCell_ (const MultiIndex< 6 > &idx) throw (concepts::MissingFeature) |
virtual void | createCell_ (const MultiIndex< 8 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 1 > &idx) |
Creation of topological entities. More... | |
virtual void | createEntity_ (const MultiIndex< 2 > &idx) |
virtual void | createEntity_ (const MultiIndex< 3 > &idx) throw (concepts::MissingFeature) |
Creation of topological entities. More... | |
virtual void | createEntity_ (const MultiIndex< 4 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 6 > &idx) throw (concepts::MissingFeature) |
virtual void | createEntity_ (const MultiIndex< 8 > &idx) throw (concepts::MissingFeature) |
void | import_ () |
Reads and builds the mesh. More... | |
virtual std::ostream & | info (std::ostream &os) const |
Returns information in an output stream. More... | |
template<uint dim> | |
void | newCell_ (MultiIndex< dim > i) |
Creates topological entities and a geometrical cell. More... | |
void | readAttributes_ (const std::string &bound, const Array< bool > &dimensions) |
void | readCells_ (const std::string &elms, const Array< bool > &dimensions, const std::vector< Real3d > &vertices) |
Reads the file with cell information and creates topological entities and geometrical cells. More... | |
uint | readInts_ (const std::string &i, std::vector< int > &v) const |
Reads integers from the string. More... | |
template<class F > | |
uint | readLine_ (const std::string &i, Array< F > &a, bool first=false) const |
Reads line of numbers from the string. More... | |
uint | readVertexLine_ (const std::string &i, uint *idx, Real3d *coord) const |
virtual void | readVertices_ () |
reads the coordinates of the vertices More... | |
Protected Attributes | |
std::vector< Cell2 * > | cell_ |
List of cells. More... | |
std::vector< Connector2 * > | cntr2_ |
List of entities. More... | |
const std::string | coord_ |
File names of coordinates and cell files. More... | |
uint | dim_ |
std::vector< Connector1 * > | edg_ |
MultiArray< 2, Edge * > | Edg_ |
MultiArray< 8, Attribute > | eightAttr_ |
const std::string | elms_ |
MultiArray< 4, Attribute > | fourAttr_ |
const uint | idxStart_ |
Starting point of indices in the files (1 or 0) More... | |
MultiArray< 1, Attribute > | oneAttr_ |
Attributes of entities. More... | |
MultiArray< 4, Quad * > | Quad_ |
MultiArray< 6, Attribute > | sixAttr_ |
MultiArray< 3, Attribute > | threeAttr_ |
MultiArray< 3, Triangle * > | Tri_ |
Array of the entities. More... | |
MultiArray< 2, Attribute > | twoAttr_ |
std::vector< Real3d > | vertices_ |
Coordinates of the vertices. More... | |
std::vector< Vertex * > | vtx_ |
List of entities. More... | |
MultiArray< 1, Vertex * > | Vtx_ |
Array of the entities. More... | |
Detailed Description
- Examples
- hpFEM2d-simple.cc, hpFEM2d.cc, inhomDirichletBCs.cc, inhomDirichletBCsLagrange.cc, inhomNeumannBCs.cc, parallelizationTutorial.cc, and RobinBCs.cc.
Definition at line 103 of file meshImport2D.hh.
Constructor & Destructor Documentation
◆ Import2dMesh() [1/2]
concepts::Import2dMesh::Import2dMesh | ( | const std::string | coord, |
const std::string | elms, | ||
const std::string | boundary, | ||
const uint | idxStart = 1 |
||
) |
Constructor.
Reads the data from three files and creates a mesh from it. The vertices, edges and cells should be number in striclty increasing order, ie. 1, 2, 3 etc.
- Parameters
-
coord File with coordinates of vertices. Format: <node> <x> <y>
or<node> <x> <y> <z>
For instance:1 1.0 1.0 2 0.0 0.5 3 0.0 0.0 4 -1.0 2.0
or1 1.0 1.0 0.0 2 0.0 0.5 0.5 3 0.0 0.0 1.0 4 -1.0 2.0 0.5
elms File with node numbers of triangles or quads. Format: <triangle> <node0> <node1> <node2>
or<quad> <node0> <node1> <node2> <node3>
For instance:1 1 2 3
or1 1 2 3 4
boundary File with node numbers of edges with boundary conditions. Format: <edge> <node0> <node1> <attr>
For instance:1 1 3 0
idxStart Starting point of indices in the files (1 or 0)
◆ Import2dMesh() [2/2]
concepts::Import2dMesh::Import2dMesh | ( | const std::string | coord, |
const std::string | elms, | ||
const uint | idxStart = 1 |
||
) |
Constructor.
Reads the data from two files and creates a mesh from it. The vertices, edges and cells should be number in strictly increasing order, ie. 1, 2, 3 etc.
- Parameters
-
coord File with coordinates of vertices. Format: <node> <x> <y>
or<node> <x> <y> <z>
For instance:1 1.0 1.0 2 0.0 0.5 3 0.0 0.0 4 -1.0 2.0
or1 1.0 1.0 0.0 2 0.0 0.5 0.5 3 0.0 0.0 1.0 4 -1.0 2.0 0.5
elms File with node numbers of triangles or quads. Format: <triangle> <node0> <node1> <node2>
or<quad> <node0> <node1> <node2> <node3>
For instance:1 1 2 3
or1 1 2 3 4
idxStart Starting point of indices in the files (1 or 0)
◆ ~Import2dMesh()
|
inlinevirtual |
Definition at line 169 of file meshImport2D.hh.
Member Function Documentation
◆ attributes_()
|
protectedinherited |
Reads attributes from file boundary
.
◆ clear_()
|
protectedinherited |
Deletes the content of field
of pointers.
Definition at line 141 of file meshImport.hh.
◆ createCell_() [1/5]
|
protectedvirtualinherited |
Creation of geometrical cells with element mapping.
Has to be implemented in derivated classes.
◆ createCell_() [2/5]
|
protectedvirtualinherited |
Creation of geometrical cells with element mapping.
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
◆ createCell_() [3/5]
|
protectedvirtualinherited |
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
◆ createCell_() [4/5]
|
protectedvirtualinherited |
◆ createCell_() [5/5]
|
protectedvirtualinherited |
◆ createEntity_() [1/6]
|
inlineprotectedvirtualinherited |
Creation of topological entities.
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
Definition at line 62 of file meshImport2D.hh.
◆ createEntity_() [2/6]
|
inlineprotectedvirtualinherited |
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
Definition at line 64 of file meshImport2D.hh.
◆ createEntity_() [3/6]
|
protectedvirtualinherited |
Creation of topological entities.
Has to be implemented in derivated classes.
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
◆ createEntity_() [4/6]
|
protectedvirtualinherited |
Reimplemented from concepts::ImportMesh.
Reimplemented in concepts::Import2dMeshGeneral.
◆ createEntity_() [5/6]
|
protectedvirtualinherited |
◆ createEntity_() [6/6]
|
protectedvirtualinherited |
◆ import_()
|
protectedinherited |
Reads and builds the mesh.
◆ info()
|
protectedvirtual |
Returns information in an output stream.
Reimplemented from concepts::Import2dMeshBase.
◆ ncell()
|
inlinevirtualinherited |
Returns the number of cells in the mesh.
Implements concepts::Mesh.
Definition at line 37 of file meshImport2D.hh.
◆ newCell_()
|
protectedinherited |
Creates topological entities and a geometrical cell.
◆ readAttributes_()
|
protectedinherited |
◆ readCells_()
|
protectedinherited |
Reads the file with cell information and creates topological entities and geometrical cells.
◆ readInts_()
|
protectedinherited |
Reads integers from the string.
- Returns
- Number of integers read
◆ readLine_()
|
protectedinherited |
Reads line of numbers from the string.
- Parameters
-
i String a resulting array of integers first flag, if the first integer should be taken into the array
- Returns
- Number of integers read
Definition at line 123 of file meshImport.hh.
◆ readVertexLine_()
|
protectedinherited |
◆ readVertices_()
|
protectedvirtualinherited |
reads the coordinates of the vertices
◆ scan()
|
virtualinherited |
Returns a scanner over the cells of the mesh.
- Postcondition
- The scanner must be deleted after usage. This has to be done by the user.
Implements concepts::Mesh2.
Member Data Documentation
◆ cell_
|
protectedinherited |
List of cells.
Definition at line 46 of file meshImport2D.hh.
◆ cntr2_
|
protectedinherited |
List of entities.
Definition at line 56 of file meshImport2D.hh.
◆ coord_
|
protectedinherited |
File names of coordinates and cell files.
Definition at line 49 of file meshImport2D.hh.
◆ dim_
|
protectedinherited |
Definition at line 53 of file meshImport2D.hh.
◆ edg_
|
protectedinherited |
Definition at line 47 of file meshImport.hh.
◆ Edg_
|
protectedinherited |
Definition at line 50 of file meshImport.hh.
◆ eightAttr_
|
protectedinherited |
Definition at line 58 of file meshImport.hh.
◆ elms_
|
protectedinherited |
Definition at line 49 of file meshImport2D.hh.
◆ fourAttr_
|
protectedinherited |
Definition at line 56 of file meshImport.hh.
◆ idxStart_
|
protectedinherited |
Starting point of indices in the files (1 or 0)
Definition at line 43 of file meshImport.hh.
◆ oneAttr_
|
protectedinherited |
Attributes of entities.
Definition at line 53 of file meshImport.hh.
◆ Quad_
|
protectedinherited |
Definition at line 59 of file meshImport2D.hh.
◆ sixAttr_
|
protectedinherited |
Definition at line 57 of file meshImport.hh.
◆ threeAttr_
|
protectedinherited |
Definition at line 55 of file meshImport.hh.
◆ Tri_
|
protectedinherited |
Array of the entities.
Definition at line 58 of file meshImport2D.hh.
◆ twoAttr_
|
protectedinherited |
Definition at line 54 of file meshImport.hh.
◆ vertices_
|
protectedinherited |
Coordinates of the vertices.
Definition at line 52 of file meshImport2D.hh.
◆ vtx_
|
protectedinherited |
List of entities.
Definition at line 46 of file meshImport.hh.
◆ Vtx_
|
protectedinherited |
Array of the entities.
Definition at line 49 of file meshImport.hh.
The documentation for this class was generated from the following file:
- geometry/meshImport2D.hh